char*转wchar*
时间: 2023-09-08 09:09:23 浏览: 163
要将char*转换为wchar*,可以使用MultiByteToWideChar函数。这个函数将一个多字节字符转换为一个宽字符。
以下是一个示例代码:
```
#include <Windows.h>
wchar_t* charToWChar(const char* str)
{
int length = MultiByteToWideChar(CP_UTF8, 0, str, -1, NULL, 0);
wchar_t* wstr = new wchar_t[length];
MultiByteToWideChar(CP_UTF8, 0, str, -1, wstr, length);
return wstr;
}
int main()
{
char* str = "Hello, world!";
wchar_t* wstr = charToWChar(str);
// use wstr
delete[] wstr;
return 0;
}
```
这个示例使用UTF-8编码将char*字符串转换为wchar_t*字符串。它首先调用MultiByteToWideChar函数获取转换后的字符串长度,然后使用new运算符为wchar_t*字符串分配内存,并调用MultiByteToWideChar函数进行实际的转换。最后,记得在使用完wchar_t*字符串后使用delete[]运算符释放内存。
相关问题
CHAR*转WCHAR*
CHAR*和WCHAR*都是C++中的字符指针类型,它们分别用于表示不同编码的字符。CHAR通常指的是ASCII字符集,而WCHAR通常指的是宽字符(WChar)。在C++中,这两种类型的转换可以通过标准库函数来完成。
要将CHAR*转换为WCHAR*,可以使用C++标准库中的std::wstring_convert类。该类提供了一个静态方法std::wstring_convert::from_chars(),可以将CHAR*转换为WCHAR*。下面是一个示例代码:
```cpp
#include <locale>
#include <codecvt>
// CHAR*源字符串
char source[256];
// 将CHAR*转换为WCHAR*
std::wstring_convert<std::codecvt_utf8<wchar_t>> converter;
wchar_t dest[256];
std::wstring ws = converter.from_bytes(std::string(source));
// 将WCHAR*复制到目标字符串中
std::wstring_adapter adapter(ws);
std::wcout << adapter.c_str() << std::endl;
```
在上面的示例中,我们首先定义了一个CHAR*源字符串,然后使用std::wstring_convert类将其转换为WCHAR*。我们使用std::codecvt_utf8<wchar_t>作为转换规则,它可以将UTF-8编码的CHAR*转换为宽字符。最后,我们将转换后的宽字符字符串复制到目标字符串中并输出。
请注意,上述示例中的代码仅适用于UTF-8编码的CHAR*字符串。如果源字符串使用其他编码格式,您需要使用适当的转换规则进行转换。此外,您还需要确保目标字符串有足够的空间来容纳转换后的宽字符字符串。
char*转WCHAR*
在C++中,当你需要将`char*`类型的字符串转换为`WCHAR*`(宽字符指针,用于表示Unicode字符串)时,你需要考虑到字符集的不同以及编码的转换。因为`char*`通常对应的是单字节字符(如ASCII),而`WCHAR*`代表的是双字节的Unicode字符。
以下是一个简单的示例:
```cpp
#include <codecvt> // 引入用于字符编码转换的头文件
#include <locale>
#include <string>
std::wstring_convert<std::codecvt_utf8<wchar_t>> conv;
// 假设有一个char*类型的字符串
const char* utf8String = "你好,世界!";
// 创建一个std::wstring对象,通过convert函数将char*转换为宽字符*
std::wstring utf16String = conv.from_bytes(utf8String);
// 现在utf16String就是对应的宽字符形式了
WCHAR* wideCharString = new WCHAR[utf16String.length() + 1]; // 要加1以包含终止符'\0'
wcscpy_s(wideCharString, utf16String.c_str());
阅读全文
相关推荐
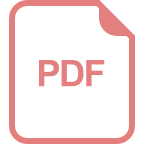
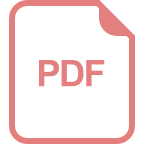
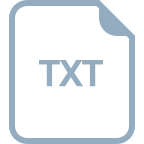













