python split numpy
时间: 2023-10-05 08:11:55 浏览: 42
In NumPy, you can split an array into multiple sub-arrays using the `numpy.split()` function. This function takes three arguments: the array to be split, the number of equally-sized sub-arrays to create, and the axis along which to split the array.
Here's an example of splitting a NumPy array using `numpy.split()`:
```python
import numpy as np
# Create a 1-dimensional array
arr = np.array([1, 2, 3, 4, 5, 6])
# Split the array into 3 sub-arrays
sub_arrays = np.split(arr, 3)
# Print the sub-arrays
for sub_arr in sub_arrays:
print(sub_arr)
```
Output:
```
[1 2]
[3 4]
[5 6]
```
In this example, the `arr` array is split into 3 equally-sized sub-arrays along the default axis 0. Each sub-array is printed separately.
You can also split a multidimensional array by specifying the axis along which to split. For example, to split a 2D array into two sub-arrays along axis 1:
```python
import numpy as np
# Create a 2-dimensional array
arr = np.array([[1, 2, 3], [4, 5, 6]])
# Split the array into 2 sub-arrays along axis 1
sub_arrays = np.split(arr, 2, axis=1)
# Print the sub-arrays
for sub_arr in sub_arrays:
print(sub_arr)
```
Output:
```
[[1 2]
[4 5]]
[[3]
[6]]
```
In this case, the `arr` array is split into two sub-arrays along axis 1. The resulting sub-arrays are printed separately.
相关推荐
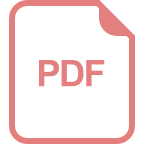
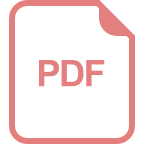
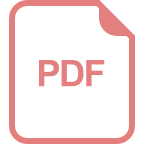














