用c语言编写程序,输入一行字符,统计其中单词的个数。这里连续的字母构成单词,单词与单词之间用空格间隔,遇到其他无效字符可视为无效输入
时间: 2024-09-27 11:16:50 浏览: 40
在 C 语言中,你可以使用标准库函数来编写一个程序来统计字符串中的单词数。下面是一个简单的示例程序,它会分割字符串并计算单词的数量:
```c
#include <stdio.h>
#include <ctype.h> // 包含 isalpha 函数
int count_words(char *str) {
int word_count = 0;
int in_word = 0; // 标记当前是否在单词内
while (*str != '\0') { // 遍历字符串直到结束
if (isalpha(*str)) { // 如果字符是字母
if (!in_word) { // 如果不在单词中,开始新单词
word_count++;
in_word = 1;
}
} else if (*str == ' ') { // 遇到空格,表示单词结束
in_word = 0;
} else { // 非字母非空格字符不算单词
continue;
}
str++; // 移动指针到下一个字符
}
// 检查最后一个单词(处理字符串结尾可能是单词的情况)
if (in_word) {
word_count++;
}
return word_count;
}
int main() {
char input[100];
printf("请输入一行字符(空格分隔单词):");
fgets(input, sizeof(input), stdin); // 读取用户输入
// 删除换行符
if (input[strlen(input)-1] == '\n') {
input[strlen(input)-1] = '\0';
}
int word_count = count_words(input);
printf("包含的单词总数: %d\n", word_count);
return 0;
}
```
在这个程序中,`count_words` 函数逐个检查输入的字符,如果是字母则认为在单词中,遇到空格或非字母字符则认为单词结束。`main` 函数用于获取用户输入,并调用 `count_words` 进行计数。
相关推荐
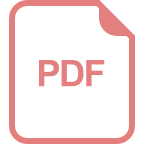
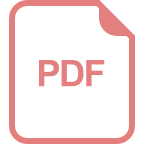














