用c语言完善简单科学计算器程序,要求尽可能利用所学过的分支判断结构语句,循环控制语句,函数和递归函数尽可能多的实现除了单项简单加减乘除之外的:复合四则运算,累加,累乘,平方,开方等运算。
时间: 2024-12-21 11:29:14 浏览: 7
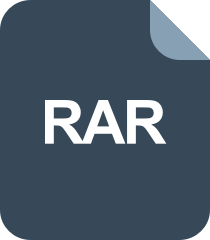
jisuanqi(CPP),好玩的c语言源码雪花,c语言程序
在C语言中,我们可以创建一个完善的科学计算器程序,它不仅包含基本的加、减、乘、除运算,还包含了复合四则运算、累加、累乘、平方和开方等高级功能。这里是一个简单的示例:
```c
#include <stdio.h>
#include <math.h>
// 单项运算函数
double add(double a, double b) {
return a + b;
}
double subtract(double a, double b) {
return a - b;
}
double multiply(double a, double b) {
return a * b;
}
double divide(double a, double b) {
if (b != 0)
return a / b;
else
printf("Error: Division by zero is not allowed.\n");
return 0; // 返回0避免悬空指针
}
// 复合运算函数(如:a*(b+c))
double compound_operation(double a, double b, char op1, char op2) {
double intermediate = 0;
switch (op1) {
case '+':
intermediate = add(a, b);
break;
case '-':
intermediate = subtract(a, b);
break;
default:
printf("Invalid operation.\n");
return 0;
}
switch (op2) {
case '*':
return multiply(intermediate, b);
case '/':
return divide(intermediate, b);
case '^': // 幂运算
return pow(intermediate, b); // 注意:实际项目需要处理负数开方
default:
printf("Invalid operation.\n");
return 0;
}
}
// 累加和累乘
double sum(int n, double arr[]) {
double total = 0;
for (int i = 0; i < n; i++) {
total += arr[i];
}
return total;
}
double product(int n, double arr[]) {
double result = 1;
for (int i = 0; i < n; i++) {
result *= arr[i];
}
return result;
}
// 平方和开方(使用数学库函数)
double square(double num) {
return pow(num, 2);
}
double sqrt(double num) {
if (num >= 0)
return sqrt(num);
else
printf("Error: Square root of negative number is not defined.\n");
return 0;
}
int main() {
double a, b;
char op1, op2;
printf("Enter two numbers and an operator (+, -, *, /, ^): ");
scanf("%lf %lf %c %c", &a, &b, &op1, &op2);
// 根据用户输入调用相应的计算函数
switch (op1) {
case '+':
printf("Result: %.2f\n", add(a, b));
break;
case '-':
printf("Result: %.2f\n", subtract(a, b));
break;
case '*':
printf("Result: %.2f\n", multiply(a, b));
break;
case '/':
printf("Result: %.2f\n", divide(a, b));
break;
case '^':
printf("Result: %.2f\n", compound_operation(a, b, op1, op2));
break;
}
int n;
printf("Enter the number of elements in array for sum or product: ");
scanf("%d", &n);
double arr[n];
printf("Enter array elements: ");
for (int i = 0; i < n; i++) {
scanf("%lf", &arr[i]);
}
printf("Sum: %.2f, Product: %.2f\n", sum(n, arr), product(n, arr));
printf("Square of %.2f: %.2f\n", b, square(b));
printf("Square root of %.2f: %.2f\n", b, sqrt(b));
return 0;
}
```
阅读全文
相关推荐
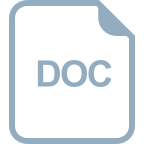
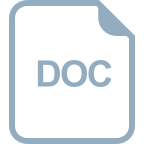

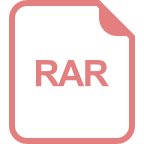
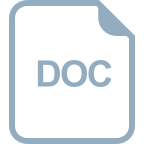
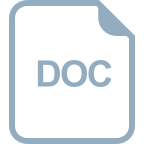
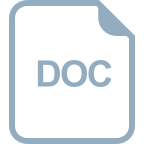
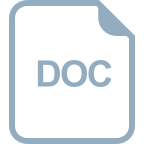
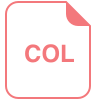


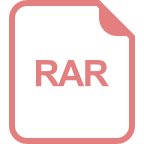
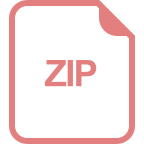
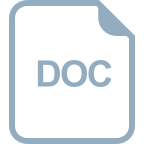
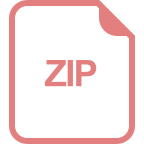
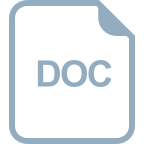
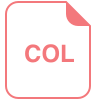
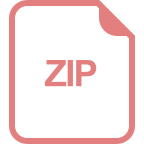
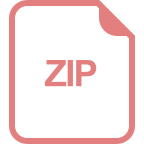