能编写一个使用STM32驱动WS2812B实现RGB流水灯的程序吗?
时间: 2024-04-15 08:27:31 浏览: 244
当然可以!下面是一个使用STM32驱动WS2812B实现RGB流水灯的简单程序示例(使用STM32Cube HAL库):
```c
#include "main.h"
#define LED_COUNT 16
uint8_t leds[LED_COUNT * 3]; // 存储RGB值的数组
// 发送一个bit
void sendBit(GPIO_TypeDef* GPIOx, uint16_t GPIO_Pin, uint8_t bit) {
if (bit) {
GPIOx->BSRR = GPIO_Pin;
__NOP(); // 等待一段时间
__NOP();
__NOP();
GPIOx->BRR = GPIO_Pin;
} else {
GPIOx->BSRR = GPIO_Pin;
__NOP();
GPIOx->BRR = GPIO_Pin;
__NOP();
__NOP();
}
}
// 发送一个字节
void sendByte(GPIO_TypeDef* GPIOx, uint16_t GPIO_Pin, uint8_t byte) {
for (uint8_t i = 0; i < 8; i++) {
sendBit(GPIOx, GPIO_Pin, byte & 0x80);
byte <<= 1;
}
}
// 发送一个LED的RGB值
void sendLED(GPIO_TypeDef* GPIOx, uint16_t GPIO_Pin, uint8_t r, uint8_t g, uint8_t b) {
sendByte(GPIOx, GPIO_Pin, g);
sendByte(GPIOx, GPIO_Pin, r);
sendByte(GPIOx, GPIO_Pin, b);
}
// 发送所有LED的RGB值
void sendLEDs(GPIO_TypeDef* GPIOx, uint16_t GPIO_Pin) {
for (uint8_t i = 0; i < LED_COUNT; i++) {
uint8_t r = leds[i * 3];
uint8_t g = leds[i * 3 + 1];
uint8_t b = leds[i * 3 + 2];
sendLED(GPIOx, GPIO_Pin, r, g, b);
}
}
int main(void) {
// 初始化代码
while (1) {
// 流水灯效果示例:依次点亮每个LED为红色
for (uint8_t i = 0; i < LED_COUNT; i++) {
for (uint8_t j = 0; j < LED_COUNT; j++) {
if (i == j) {
leds[j * 3] = 255; // 红色分量设置为最大值
leds[j * 3 + 1] = 0; // 绿色分量设置为最小值
leds[j * 3 + 2] = 0; // 蓝色分量设置为最小值
} else {
leds[j * 3] = 0;
leds[j * 3 + 1] = 0;
leds[j * 3 + 2] = 0;
}
}
sendLEDs(GPIOx, GPIO_Pin); // 发送LED的RGB值
HAL_Delay(100); // 延时一段时间
}
}
}
```
请注意,上述代码只是一个示例,你需要根据自己的硬件连接和需求进行适当的修改。具体的引脚连接和配置等细节,可以参考STM32的官方文档和WS2812B的数据手册。
阅读全文
相关推荐
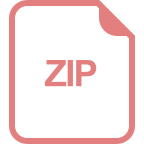
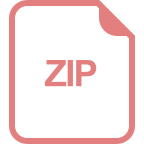
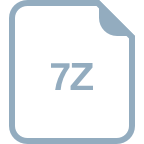


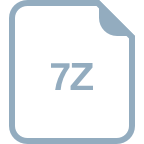
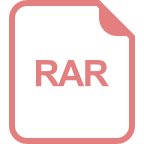
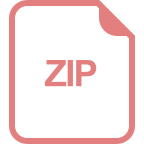
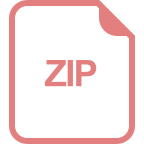
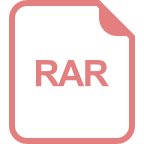
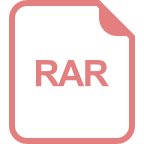
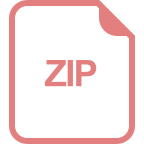
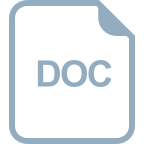
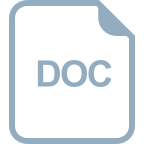
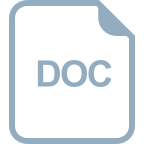

