实现在指定位置插入元素的算法int Insert_Linked_Node(Linked_Node *L, int i, elemtype data),在单链表L的第i个位置(i为逻辑位置)加入数据元素data,并返回插入操作是否成功(返回0表示成功,返回-1表示不成功)
时间: 2024-10-28 13:08:13 浏览: 28
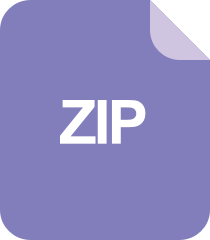
circular_linked_list_4_6.zip_Linked list
要在单链表`L`的指定位置`i`插入元素,我们需要创建一个新的节点,并将它插入到现有链表中。如果`i`小于1或大于链表的实际长度,那么插入操作不成功,应该返回-1。以下是C语言的一个简单实现:
```c
struct Linked_Node {
int data;
struct Linked_Node* next;
};
int Insert_Linked_Node(struct Linked_Node **L, int i, int data) {
// 初始条件:新节点已创建,插入位置越界检查
if (*L == NULL || i <= 0) return -1;
// 创建新节点
struct Linked_Node* newNode = (struct Linked_Node*)malloc(sizeof(struct Linked_Node));
newNode->data = data;
newNode->next = NULL;
// 插入位置为头节点时,直接连接新旧节点
if (i == 1) {
newNode->next = *L;
*L = newNode;
return 0;
}
// 查找插入位置
struct Linked_Node* current = *L;
int position = 1;
while (current && position < i - 1) {
current = current->next;
position++;
}
// 如果找到了正确的插入位置,插入新节点
if (current) {
newNode->next = current->next;
current->next = newNode;
return 0;
} else {
// 插入位置超出链表长度,返回错误
return -1;
}
}
阅读全文
相关推荐
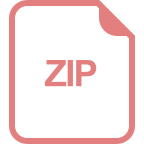
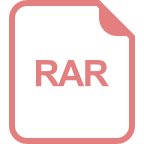





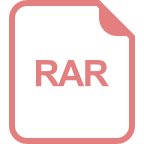
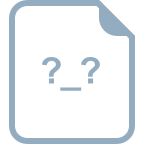
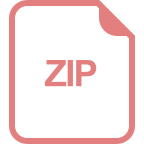
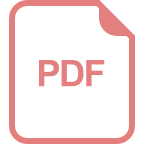
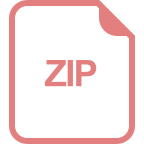
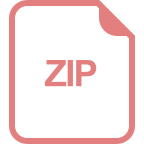
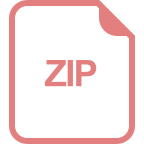
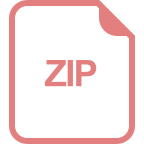



