用C语言编写代码解决矩阵的线性运算
时间: 2024-10-25 07:02:04 浏览: 43
在C语言中,我们可以创建函数来处理矩阵的线性运算,比如加法、减法和乘法。这里给出一个简单的例子,展示如何实现两个整数矩阵的加法:
```c
#include <stdio.h>
// 定义矩阵结构体
typedef struct {
int rows;
int cols;
int data[100][100]; // 假设最大矩阵大小为10x10
} Matrix;
// 矩阵加法函数
Matrix add_matrices(Matrix a, Matrix b) {
if (a.rows != b.rows || a.cols != b.cols) {
printf("Error: Matrices must have the same dimensions.\n");
return a; // 返回原矩阵以避免错误
}
Matrix result = {a.rows, a.cols};
for (int i = 0; i < a.rows; ++i) {
for (int j = 0; j < a.cols; ++j) {
result.data[i][j] = a.data[i][j] + b.data[i][j];
}
}
return result;
}
// 打印矩阵
void print_matrix(Matrix m) {
for (int i = 0; i < m.rows; ++i) {
for (int j = 0; j < m.cols; ++j) {
printf("%d ", m.data[i][j]);
}
printf("\n");
}
}
int main() {
Matrix matrix1 = {3, 3, {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}}};
Matrix matrix2 = {3, 3, {{9, 8, 7}, {6, 5, 4}, {3, 2, 1}}};
Matrix sum = add_matrices(matrix1, matrix2);
printf("Sum of matrices:\n");
print_matrix(sum);
return 0;
}
```
在这个示例中,`add_matrices`函数接受两个矩阵作为输入,检查它们是否具有相同的行和列,然后逐元素相加。`print_matrix`函数用于显示矩阵。
阅读全文
相关推荐
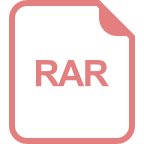
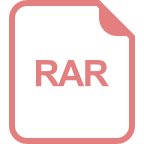
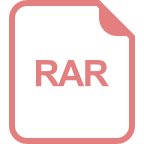















