使用numpy.interp对二维坐标点根据时间做线性插值
时间: 2024-02-15 11:02:10 浏览: 21
numpy.interp只能用于一维数组的线性插值,无法直接对二维坐标点进行插值。但是,我们可以分别对坐标点的x和y坐标进行插值,然后将插值结果组合成新的坐标点。
下面是一个示例代码,假设我们有一个二维坐标点列表coords,其中每个坐标点有x、y和t三个属性,需要对其根据时间t做线性插值。
```python
import numpy as np
# 原始坐标点列表
coords = [
{'x': 0, 'y': 0, 't': 0},
{'x': 1, 'y': 2, 't': 1},
{'x': 2, 'y': 4, 't': 2},
{'x': 3, 'y': 6, 't': 3},
{'x': 4, 'y': 8, 't': 4},
]
# 提取x、y和t坐标作为一维数组
x = np.array([coord['x'] for coord in coords])
y = np.array([coord['y'] for coord in coords])
t = np.array([coord['t'] for coord in coords])
# 生成新的时间序列
new_t = np.linspace(0, 4, num=9, endpoint=True)
# 对x和y坐标分别进行插值
new_x = np.interp(new_t, t, x)
new_y = np.interp(new_t, t, y)
# 将插值结果组合成新的坐标点
new_coords = [{'x': new_x[i], 'y': new_y[i], 't': new_t[i]} for i in range(len(new_t))]
print(new_coords)
```
在上述代码中,我们首先从原始坐标点列表中提取出x、y和t坐标,然后使用numpy.interp对x和y坐标进行线性插值。最后,我们将插值结果组合成新的坐标点列表new_coords,其中每个坐标点的x、y和t坐标都是根据时间t进行线性插值得到的。
相关推荐
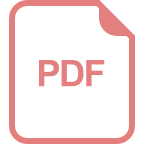
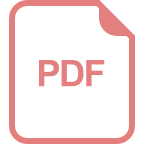














