mongoTemplate.findAndModify和mongoTemplate.upsert
时间: 2024-05-26 11:16:44 浏览: 13
`mongoTemplate.findAndModify()`和`mongoTemplate.upsert()`是Spring Data MongoDB提供的两个常用的方法。
`mongoTemplate.findAndModify()`方法用于查找并修改文档。可以指定查询条件、修改数据和返回结果类型等参数。该方法会返回修改之前的文档或修改之后的文档,取决于传入的参数。
`mongoTemplate.upsert()`方法用于更新或插入数据。如果指定的查询条件匹配到了一条文档,则会更新这个文档;否则会插入一条新的文档。可以指定更新的数据和查询条件等参数。
在使用这两个方法时需要注意以下几点:
1. `mongoTemplate.findAndModify()`方法在修改文档时会锁定整个文档,因此在高并发场景下可能会影响性能。需要根据具体情况选择是否使用该方法。
2. `mongoTemplate.upsert()`方法在插入新文档时会自动添加一个`_id`字段,如果已经存在该字段则会更新相应的文档。
3. 由于`mongoTemplate.upsert()`方法默认只会更新第一条匹配到的文档,因此需要注意查询条件是否足够准确,否则可能会更新不正确的文档。
总之,这两个方法都是MongoDB中比较常用的操作,可以帮助我们更方便地进行文档的修改和插入。
相关问题
mongotemplate.upsert
### 回答1:
mongotemplate.upsert是MongoTemplate类中的一个方法,用于在MongoDB中执行更新操作。如果指定的文档不存在,则会插入一个新文档。如果指定的文档已经存在,则会更新该文档。这个方法的作用类似于SQL中的“插入或更新”操作。
### 回答2:
mongotemplate.upsert是Spring Data MongoDB提供的方法,用于执行一个upsert操作。upsert是指在执行更新操作时,如果记录不存在,则会插入一条新记录。
在MongoDB中,upsert操作可以使用$setOnInsert操作符来指定插入的值。当使用mongotemplate.upsert方法时,如果满足查询条件的记录存在,则会执行更新操作,更新的内容是通过$set操作符来指定的。如果满足查询条件的记录不存在,则会执行插入操作,插入的内容是通过$setOnInsert操作符来指定的。
例如,假设我们有一个名为users的集合,其中的记录格式如下:
{
"_id": ObjectId("60c7e8fd387bb5ae091b688a"),
"name": "John",
"age": 25,
"address": "123 Main St"
}
我们可以使用mongotemplate.upsert方法来更新或插入记录。比如,我们想更新name为"John"的记录,将age字段增加1:
Query query = new Query(Criteria.where("name").is("John"));
Update update = new Update().inc("age", 1);
mongotemplate.upsert(query, update, Users.class);
如果存在name为"John"的记录,则会执行更新操作,结果为:
{
"_id": ObjectId("60c7e8fd387bb5ae091b688a"),
"name": "John",
"age": 26,
"address": "123 Main St"
}
如果不存在name为"John"的记录,则会执行插入操作,结果为:
{
"_id": ObjectId("60c7e8fd387bb5ae091b688a"),
"name": "John",
"age": 1
}
这就是mongotemplate.upsert方法的用法和作用。它可以方便地执行upsert操作,根据条件进行更新或插入操作,提高了开发效率。
### 回答3:
mongotemplate.upsert是MongoDB中的一个操作方法,用于在集合中插入或更新数据。如果指定的查询条件在集合中找到匹配记录,则会进行更新操作,如果找不到匹配记录,则会进行插入操作。这个方法是根据指定的查询条件,先在集合中进行查询,然后根据查询结果来判断是插入数据还是更新数据。
在使用mongotemplate.upsert方法时,首先需要创建一个更新对象,用于指定需要更新的字段和值。然后创建一个查询对象,用于指定查询条件。最后将更新对象和查询对象作为参数传递给mongotemplate.upsert方法。
在进行upsert操作时,如果集合中存在满足查询条件的记录,则会将更新对象中的字段值更新到该记录中。如果集合中不存在满足查询条件的记录,则会将更新对象中的字段值插入到集合中,成为一条新的记录。
使用mongotemplate.upsert方法可以方便地实现根据不同的查询条件进行插入或更新操作。这个方法在处理大量数据时尤其有用,可以避免查询和更新操作分开进行,提高了效率。
综上所述,mongotemplate.upsert是MongoDB中的一个操作方法,用于在集合中进行插入或更新操作,根据指定的查询条件来判断操作类型。使用这个方法可以方便地实现插入或更新操作,并提高效率。
mongotemplate.upsert用法
`MongoTemplate`'s `upsert` method is used to perform an "upsert" operation in MongoDB. "Upsert" is a combination of "update" and "insert" operations. It updates an existing document if it matches the query criteria, or inserts a new document if no matching document is found.
Here's an example of how to use the `upsert` method in `MongoTemplate`:
```java
import org.springframework.data.mongodb.core.MongoTemplate;
import org.springframework.data.mongodb.core.query.Criteria;
import org.springframework.data.mongodb.core.query.Query;
import org.springframework.data.mongodb.core.query.Update;
public class UpsertExample {
private MongoTemplate mongoTemplate;
public void upsertDocument(String id, String name, int age) {
// Create a query to find the document by id
Query query = new Query(Criteria.where("_id").is(id));
// Create an update object with the new values
Update update = new Update();
update.set("name", name);
update.set("age", age);
// Perform the upsert operation
mongoTemplate.upsert(query, update, YourDocumentClass.class);
}
}
```
In this example, we have a method called `upsertDocument` that performs an upsert operation. It takes an `id`, `name`, and `age` as parameters. First, we create a `Query` object to find the document based on the specified `id`. Then, we create an `Update` object with the new values for `name` and `age`. Finally, we call the `upsert` method on `MongoTemplate` with the query, update, and the class of the document you want to upsert.
Make sure to replace `YourDocumentClass` with the actual class representing your MongoDB document.
Note that `upsert` is just one of the many methods available in `MongoTemplate` for performing CRUD operations in MongoDB. You can refer to the Spring Data MongoDB documentation for more details on using `MongoTemplate` and other features it provides.
相关推荐
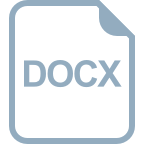












