java list多条件分组
时间: 2023-08-05 16:20:59 浏览: 73
可以通过Java 8的Stream API和Collectors.groupingBy()方法来实现多条件分组。以下是一个示例代码:
假设有一个Student类,包含id、name、age和gender四个属性。
```java
List<Student> students = // 获得学生列表
Map<String, Map<Integer, List<Student>>> resultMap = students.stream()
.collect(Collectors.groupingBy(Student::getGender, Collectors.groupingBy(Student::getAge)));
```
上述代码将学生列表按照性别和年龄进行了分组,得到了一个嵌套的Map。其中,外层Map的Key为性别,值为内层Map;内层Map的Key为年龄,值为对应年龄的学生列表。
可以根据实际需求进行修改,例如添加更多的分组条件、使用其他的聚合函数等。
相关问题
java list 分组
可以使用Java 8的Stream API来实现分组操作。可以使用Collectors.groupingBy()方法来分组,该方法接受一个Function作为分组条件,分组条件指定了如何对元素进行分组。以下是一个示例代码:
```
import java.util.*;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9);
Map<Boolean, List<Integer>> result = numbers.stream()
.collect(Collectors.partitioningBy(n -> n % 2 == 0));
System.out.println(result);
}
}
```
输出结果为:{false=[1, 3, 5, 7, 9], true=[2, 4, 6, 8]}。
在这个示例中,我们使用了Collectors.partitioningBy()方法来将数字列表分为偶数和奇数两个组。该方法也接受一个Function作为分组条件,但是该Function的返回值必须为boolean类型。在本例中,我们使用n % 2 == 0来判断数字n是偶数还是奇数。
java list stream分组值为数量
在 Java 中,可以使用 Stream API 的 `collect` 方法结合 `Collectors.groupingBy` 函数来对 List 中的数据按照某个属性进行分组,并统计每个组的数量。这个过程通常用于数据的聚合操作,比如将列表中的对象按某种条件划分到不同的组,然后得到每个组内元素的数量。
以下是一个简单的示例,假设我们有一个 Person 对象的列表,想要按照年龄 groupBy 并计算每组的人数:
```java
List<Person> people = ... // 你的Person对象列表
Map<Integer, Long> ageGroupedCount = people.stream()
.collect(Collectors.groupingBy(Person::getAge,
Collectors.counting()));
// 输出结果是一个 map,key 是年龄,value 是该年龄范围内的人数
for (Map.Entry<Integer, Long> entry : ageGroupedCount.entrySet()) {
System.out.println("年龄 " + entry.getKey() + " 的人有 " + entry.getValue() + " 个");
}
```
在这个例子中,`Person::getAge` 表示使用 `Person` 类的 `getAge()` 方法作为键生成器(KeyExtractor),`Collectors.counting()` 表示计算每个组内的元素数量。
阅读全文
相关推荐
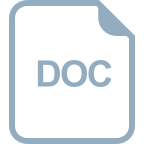
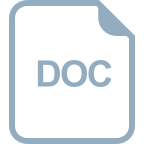
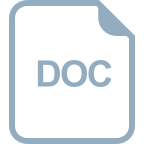
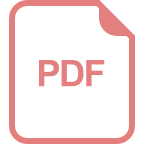




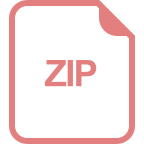







