c++ 实现 https
时间: 2023-06-29 10:09:32 浏览: 75
要使用C++实现HTTPS,你需要使用SSL/TLS协议来建立安全连接。下面是一个简单的示例代码,可以用于建立HTTPS连接并发送GET请求:
```c++
#include <iostream>
#include <string>
#include <sstream>
#include <fstream>
#include <vector>
#include <cstdlib>
#include <cstring>
#include <cstdio>
#include <fcntl.h>
#include <unistd.h>
#include <errno.h>
#include <netdb.h>
#include <arpa/inet.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <openssl/ssl.h>
#include <openssl/err.h>
using namespace std;
void print_error(const char* msg) {
perror(msg);
exit(1);
}
int main(int argc, char *argv[]) {
if (argc != 2) {
cerr << "usage: " << argv[0] << " <url>" << endl;
return 1;
}
string url = argv[1];
// 解析URL
string host, path;
int port;
if (url.substr(0, 8) == "https://") {
port = 443;
url = url.substr(8);
} else {
cerr << "error: unsupported protocol" << endl;
return 1;
}
size_t pos = url.find('/');
if (pos == string::npos) {
host = url;
path = "/";
} else {
host = url.substr(0, pos);
path = url.substr(pos);
}
// 建立TCP连接
int sockfd = socket(AF_INET, SOCK_STREAM, 0);
if (sockfd < 0) {
print_error("socket");
}
struct hostent* server = gethostbyname(host.c_str());
if (server == NULL) {
cerr << "error: no such host" << endl;
return 1;
}
struct sockaddr_in addr;
memset(&addr, 0, sizeof(addr));
addr.sin_family = AF_INET;
addr.sin_port = htons(port);
memcpy(&addr.sin_addr.s_addr, server->h_addr, server->h_length);
if (connect(sockfd, (struct sockaddr*)&addr, sizeof(addr)) < 0) {
print_error("connect");
}
// 初始化SSL
SSL_library_init();
SSL_CTX* ssl_ctx = SSL_CTX_new(TLSv1_2_client_method());
if (ssl_ctx == NULL) {
cerr << "error: failed to create SSL_CTX" << endl;
return 1;
}
SSL* ssl = SSL_new(ssl_ctx);
if (ssl == NULL) {
cerr << "error: failed to create SSL" << endl;
return 1;
}
if (SSL_set_fd(ssl, sockfd) == 0) {
cerr << "error: failed to set SSL socket" << endl;
return 1;
}
if (SSL_connect(ssl) != 1) {
cerr << "error: failed to establish SSL connection" << endl;
return 1;
}
// 发送HTTP请求
stringstream ss;
ss << "GET " << path << " HTTP/1.1\r\n";
ss << "Host: " << host << "\r\n";
ss << "Connection: close\r\n";
ss << "\r\n";
string request = ss.str();
if (SSL_write(ssl, request.c_str(), request.size()) <= 0) {
cerr << "error: failed to send HTTP request" << endl;
return 1;
}
// 读取HTTP响应
char buf[1024];
int len;
while ((len = SSL_read(ssl, buf, sizeof(buf))) > 0) {
fwrite(buf, 1, len, stdout);
}
if (len < 0) {
cerr << "error: failed to read HTTP response" << endl;
return 1;
}
// 关闭SSL和TCP连接
SSL_shutdown(ssl);
SSL_free(ssl);
SSL_CTX_free(ssl_ctx);
close(sockfd);
return 0;
}
```
需要注意的是,在编译时要链接OpenSSL库,例如:
```
g++ -o https https.cpp -lssl -lcrypto
```
相关推荐
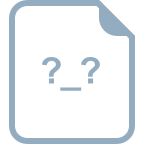
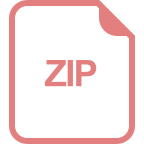














