你好 你给我提供一个 vue+vue-amap 的代码吗 代码功能为:全屏展示地图以及地物的坐标信息,能够在地图上查询相关位置 在此基础上 能够用鼠标画线闭合在地图上划定区块,标注区块的信息,对所划定区块进行面积计算。能够用鼠标选择地图上某个地点 进行标注 填写信息
时间: 2024-02-23 16:57:06 浏览: 28
好的,以下是一个简单的示例代码,包含了你所需要的功能:
```html
<template>
<div class="map-container">
<div ref="mapContainer" class="map"></div>
<div class="tools">
<button @click="drawPolygon">划定区块</button>
<button @click="clearPolygon">清除区块</button>
<button @click="measureArea">计算面积</button>
<button @click="addMarker">添加标记</button>
</div>
</div>
</template>
<script>
import AMapLoader from "@amap/amap-jsapi-loader";
export default {
name: "Map",
data() {
return {
map: null,
polygon: null,
markers: [],
infoWindow: null,
};
},
mounted() {
AMapLoader.load({
key: "your-key-here",
version: "2.0",
plugins: ["AMap.MarkerClusterer"],
}).then((AMap) => {
this.map = new AMap.Map(this.$refs.mapContainer, {
zoom: 13,
center: [116.397428, 39.90923],
});
this.infoWindow = new AMap.InfoWindow({
offset: new AMap.Pixel(0, -20),
isCustom: true,
content: "<div class='info-window'></div>",
});
this.map.on("click", (event) => {
this.addMarker(event.lnglat);
});
});
},
methods: {
drawPolygon() {
if (!this.polygon) {
this.polygon = new AMap.Polygon({
strokeWeight: 2,
fillOpacity: 0.2,
fillColor: "#0088ff",
map: this.map,
});
this.map.on("click", (event) => {
let path = this.polygon.getPath();
path.push(event.lnglat);
this.polygon.setPath(path);
});
}
},
clearPolygon() {
if (this.polygon) {
this.polygon.setMap(null);
this.polygon = null;
}
},
measureArea() {
if (this.polygon) {
let area = this.polygon.getArea() / 1000000;
alert(`面积为 ${area.toFixed(2)} 平方千米`);
}
},
addMarker(lnglat) {
let marker = new AMap.Marker({
position: lnglat,
map: this.map,
});
marker.on("click", (event) => {
let content = this.infoWindow.getContent();
content.innerHTML = `
<div>
<label>名称:</label>
<input type="text" class="name" value="">
</div>
<div>
<label>描述:</label>
<textarea class="description"></textarea>
</div>
<div>
<button class="save">保存</button>
</div>
`;
let saveButton = content.querySelector(".save");
saveButton.addEventListener("click", () => {
let name = content.querySelector(".name").value;
let description = content.querySelector(".description").value;
this.saveMarkerInfo(marker, name, description);
});
this.infoWindow.open(this.map, marker.getPosition());
});
this.markers.push(marker);
},
saveMarkerInfo(marker, name, description) {
// TODO: 保存标记信息
alert(`已保存标记:${name}`);
this.infoWindow.close();
},
},
};
</script>
<style scoped>
.map-container {
position: relative;
width: 100%;
height: 100%;
}
.map {
width: 100%;
height: 100%;
}
.tools {
position: absolute;
top: 20px;
left: 20px;
z-index: 1;
}
.info-window {
width: 200px;
height: 120px;
padding: 10px;
}
</style>
```
需要注意的是,这里使用了 `@amap/amap-jsapi-loader` 库来加载高德地图 API。你需要先安装该库并获取高德地图的 API Key 后才能运行代码。同时,你需要根据具体需求来完善代码中的 `saveMarkerInfo` 方法,将标记的名称和描述信息保存到数据库或其他地方。
相关推荐
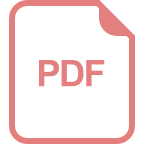
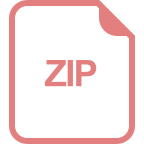
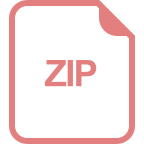














