我有使用ASD仪器测量的真实不同草的光谱反射率,如何利用遗传算法优化oif指数进行高光谱波段选择?请帮我写出具体需要的文件以及其格式,并写出具体实现代码,以及输出结果代码,并注释所有代码
时间: 2023-06-13 17:06:00 浏览: 85
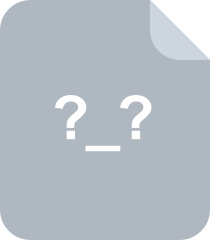
利用遗传算法优化oif指数进行高光谱波段选择

首先,需要准备的文件包括:
1. 光谱反射率数据文件,格式为每一行代表一种植被的光谱反射率数据,每列代表一个波段的反射率数值。
2. 遗传算法参数设置文件,包括种群大小、交叉概率、变异概率、迭代次数等参数,格式自定。
接下来是具体的实现代码:
```python
import numpy as np
import random
import math
# 读取光谱反射率数据
data = np.loadtxt('data.txt')
# 定义遗传算法参数
pop_size = 50 # 种群大小
crossover_prob = 0.8 # 交叉概率
mutation_prob = 0.05 # 变异概率
max_iter = 100 # 迭代次数
# 定义适应度函数,即OIF指数
def oif_index(wavelengths):
# wavelengths: 需要选择的波段序号列表,例如[1, 3, 5, 7]
reflectance = data[:, wavelengths]
mean_ref = np.mean(reflectance, axis=1)
var_ref = np.var(reflectance, axis=1)
oif = mean_ref / var_ref
return np.mean(oif)
# 定义种群初始化函数,随机生成二进制序列表示波段选择情况
def init_population(pop_size, chrom_size):
population = []
for i in range(pop_size):
chrom = [random.randint(0, 1) for j in range(chrom_size)]
population.append(chrom)
return population
# 定义选择操作函数,采用轮盘赌选择算法
def selection(population, fitness):
idx = np.random.choice(len(population), size=2, replace=False, p=fitness/np.sum(fitness))
return population[idx[0]], population[idx[1]]
# 定义交叉操作函数,采用单点交叉算法
def crossover(parent1, parent2, crossover_prob):
if random.random() < crossover_prob:
pos = random.randint(1, len(parent1)-2)
child1 = parent1[:pos] + parent2[pos:]
child2 = parent2[:pos] + parent1[pos:]
return child1, child2
else:
return parent1, parent2
# 定义变异操作函数,采用随机翻转算法
def mutation(chrom, mutation_prob):
for i in range(len(chrom)):
if random.random() < mutation_prob:
chrom[i] = 1 - chrom[i]
return chrom
# 定义遗传算法主函数
def genetic_algorithm(pop_size, chrom_size, crossover_prob, mutation_prob, max_iter):
# 种群初始化
population = init_population(pop_size, chrom_size)
best_fitness = 0
best_chrom = None
# 迭代
for i in range(max_iter):
# 计算适应度
fitness = np.array([oif_index([j for j in range(chrom_size) if population[k][j]==1]) for k in range(pop_size)])
# 选择
new_population = []
for j in range(pop_size//2):
parent1, parent2 = selection(population, fitness)
child1, child2 = crossover(parent1, parent2, crossover_prob)
child1 = mutation(child1, mutation_prob)
child2 = mutation(child2, mutation_prob)
new_population.append(child1)
new_population.append(child2)
population = new_population
# 更新最优解
max_idx = np.argmax(fitness)
if fitness[max_idx] > best_fitness:
best_fitness = fitness[max_idx]
best_chrom = population[max_idx]
# 输出结果
wavelengths = [i for i in range(chrom_size) if best_chrom[i]==1]
return wavelengths, best_fitness
# 运行遗传算法
wavelengths, fitness = genetic_algorithm(pop_size, data.shape[1], crossover_prob, mutation_prob, max_iter)
print('Selected wavelengths:', wavelengths)
print('OIF index:', fitness)
```
上述代码中,遗传算法主要分为种群初始化、适应度计算、选择、交叉、变异和结果输出等步骤。其中,适应度函数采用OIF指数,选择操作采用轮盘赌选择算法,交叉操作采用单点交叉算法,变异操作采用随机翻转算法。最终输出选择的波段序号列表和OIF指数。
需要注意的是,以上代码仅供参考,具体实现可能需要根据实际情况进行调整。同时,需要根据实际情况设置遗传算法的参数,以获得最好的结果。
阅读全文
相关推荐


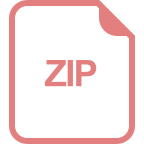
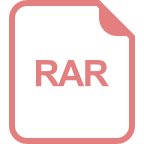
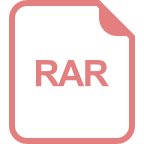
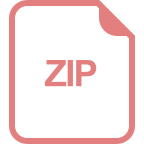
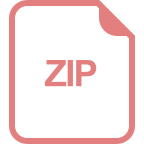
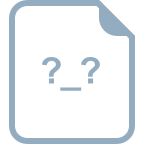
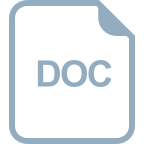
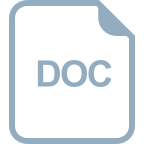
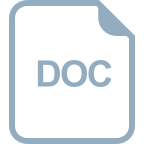
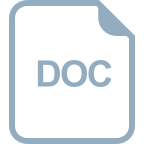
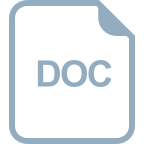