用html和css和js和es6帮我写一份粒子生长树的完整代码
时间: 2024-04-08 12:34:28 浏览: 20
当然可以帮您编写使用 HTML、CSS、JavaScript 和 ES6 的粒子生长树的完整代码。以下是一个示例:
```html
<!DOCTYPE html>
<html>
<head>
<style>
#canvas {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
}
</style>
</head>
<body>
<canvas id="canvas"></canvas>
<script>
// 使用 ES6 的类来表示粒子
class Particle {
constructor(position, parentPosition) {
this.position = position;
this.parentPosition = parentPosition;
this.children = [];
}
addChild(child) {
this.children.push(child);
}
}
// 创建画布
const canvas = document.getElementById('canvas');
const ctx = canvas.getContext('2d');
// 设置画布大小
canvas.width = window.innerWidth;
canvas.height = window.innerHeight;
// 生成粒子生长树
function generateTree(numParticles, maxDepth, growthStep) {
const particles = [new Particle({ x: canvas.width / 2, y: canvas.height }, null)];
for (let i = 0; i < maxDepth; i++) {
const newParticles = [];
for (const particle of particles) {
for (let j = 0; j < numParticles; j++) {
const deltaX = Math.random() * growthStep - growthStep / 2;
const deltaY = Math.random() * growthStep - growthStep / 2;
const newPosition = {
x: particle.position.x + deltaX,
y: particle.position.y - growthStep + deltaY
};
const newParticle = new Particle(newPosition, particle.position);
particle.addChild(newParticle);
newParticles.push(newParticle);
}
}
particles.push(...newParticles);
}
return particles;
}
// 绘制粒子生长树
function drawTree(particles) {
ctx.clearRect(0, 0, canvas.width, canvas.height);
ctx.strokeStyle = 'black';
function drawParticle(particle) {
ctx.beginPath();
ctx.moveTo(particle.parentPosition.x, particle.parentPosition.y);
ctx.lineTo(particle.position.x, particle.position.y);
ctx.stroke();
for (const child of particle.children) {
drawParticle(child);
}
}
for (const particle of particles) {
drawParticle(particle);
}
}
// 示例用法
const numParticles = 3;
const maxDepth = 5;
const growthStep = 50;
const particles = generateTree(numParticles, maxDepth, growthStep);
drawTree(particles);
</script>
</body>
</html>
```
这段代码使用了 HTML、CSS 和 JavaScript(包括 ES6)来实现粒子生长树。HTML 部分定义了一个 canvas 元素用于绘制树形结构。CSS 部分设置了 canvas 的样式,使其占满整个页面。JavaScript 部分使用 ES6 的类来表示粒子,并提供了生成和绘制粒子生长树的函数。
在 JavaScript 部分,生成粒子生长树的 `generateTree` 函数接受三个参数:`numParticles` 表示每个粒子在每一步中生成的子粒子数量,`maxDepth` 表示生长树的最大深度,`growthStep` 表示每一步中粒子的位置变化量。该函数使用循环逐步生成粒子生长树,并返回所有生成的粒子。
绘制粒子生长树的 `drawTree` 函数使用 canvas 的 API 绘制树形结构。它使用递归的方式绘制每个粒子及其子粒子之间的连线。
示例用法部分展示了如何使用上述函数生成并绘制一个简单的粒子生长树。您可以根据需要调整参数,并进一步扩展和美化界面。希望对您有所帮助!
相关推荐
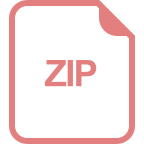
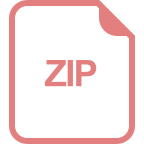
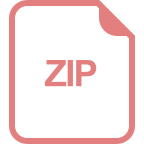














