transform.right = rb.velocity;
时间: 2024-09-12 09:01:45 浏览: 29
在Unity游戏开发中,`transform.right` 是一个用于获取游戏对象当前朝向的向量,其返回值是对象的本地右方向向量,通常用于2D和3D空间中的旋转和位置计算。`rb.velocity` 表示刚体(Rigidbody)的当前速度,包含了线性速度的信息,是一个三维向量,包含了x、y、z三个分量。
将 `transform.right` 赋值给 `rb.velocity` 的一部分(通常是在2D游戏中使用x分量或y分量),意味着我们正在设置游戏对象的运动方向,使其沿当前的右方向移动。这个操作通常用于让对象根据其当前朝向移动。
例如,如果你想要一个游戏对象沿着其自身的右方向以一定的速度移动,你可以只设置 `rb.velocity` 的x分量(在2D游戏中)或y分量(在2D游戏中,若y轴是向上方向的话)。代码可能如下所示:
```csharp
rb.velocity = new Vector2(transform.right.x * speed, rb.velocity.y);
```
在这段代码中,`speed` 是我们希望对象沿右方向移动的速度。`transform.right.x` 获取的是对象右方向的x分量,我们用这个值乘以速度来设置新的水平速度。`rb.velocity.y` 保持了原来的垂直速度分量,确保对象的垂直运动不受影响。
相关推荐
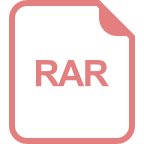
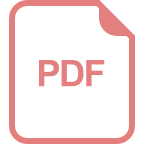
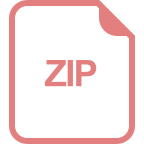
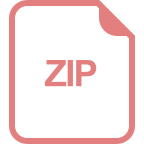
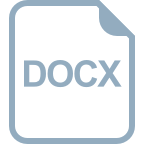
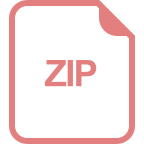
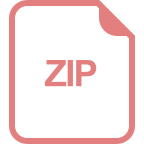
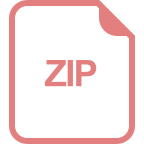
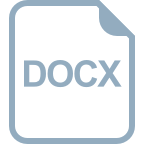
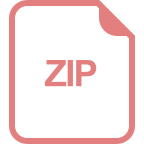