写一个动物类,动物有属性:名字,身高,体重,年龄,并将此类放到vector容器中,并对动物的体重进行排序,最后打印输出各个动物的体重,以及体重最重的动物
时间: 2023-12-08 15:04:10 浏览: 54
好的,我来为您编写一个动物类,并实现对动物体重的排序和输出:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
class Animal {
public:
Animal(std::string name, float height, float weight, int age)
: name_(name), height_(height), weight_(weight), age_(age) {}
std::string get_name() const { return name_; }
float get_height() const { return height_; }
float get_weight() const { return weight_; }
int get_age() const { return age_; }
private:
std::string name_;
float height_;
float weight_;
int age_;
};
bool compare_weight(const Animal& a, const Animal& b) {
return a.get_weight() < b.get_weight();
}
int main() {
std::vector<Animal> animals;
animals.emplace_back("Lion", 1.2f, 200.0f, 8);
animals.emplace_back("Elephant", 2.5f, 3000.0f, 20);
animals.emplace_back("Giraffe", 5.0f, 800.0f, 15);
animals.emplace_back("Tiger", 1.5f, 250.0f, 10);
std::sort(animals.begin(), animals.end(), compare_weight);
std::cout << "Sorted by weight:" << std::endl;
for (const auto& animal : animals) {
std::cout << animal.get_name() << ": " << animal.get_weight() << "kg" << std::endl;
}
std::cout << "Heaviest animal: " << animals.back().get_name() << std::endl;
return 0;
}
```
这个程序定义了一个 `Animal` 类,其中包含了名字、身高、体重和年龄等属性。然后将这些动物放入了 `std::vector` 容器中,通过 `std::sort` 函数按照体重进行排序,并输出每个动物的体重以及体重最重的动物。
阅读全文
相关推荐
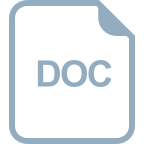
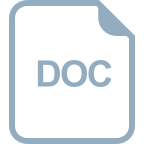
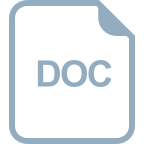
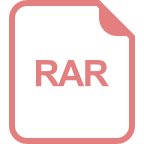
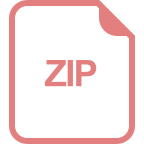
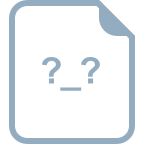
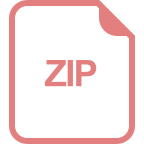
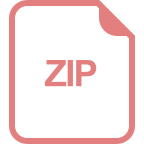
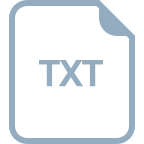
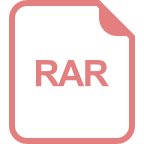
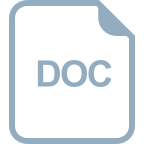
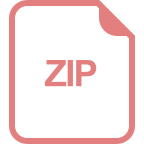
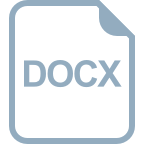
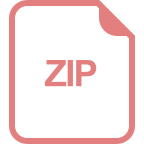
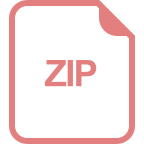
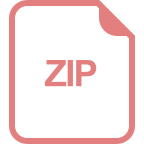