建立一个链表,存储1,2,3,4和你学号后三位数,观察内存地址变化情况。c语言
时间: 2024-09-17 11:03:30 浏览: 41
在C语言中,我们可以创建一个动态链表来存储整数,包括1、2、3、4以及你的学号后三位。首先,我们需要定义一个结构体`Node`来表示链表中的节点,包含一个整数值和指向下一个节点的指针:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int value;
struct Node* next;
} Node;
void insertNode(Node** head, int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->value = data;
newNode->next = *head;
*head = newNode;
}
int main() {
Node* head = NULL;
// 插入1
insertNode(&head, 1);
printf("Node 1 at address %p\n", head);
// 插入2
insertNode(&head, 2);
printf("Node 2 at address %p\n", head->next);
// 插入3
insertNode(&head->next, 3);
printf("Node 3 at address %p\n", head->next->next);
// 模拟学号后三位(这里假设你的学号为123)
int your_id = 123;
insertNode(&head->next->next, your_id % 1000); // 取模操作只保留最后三位
while (head != NULL) {
printf("Value: %d, Address: %p\n", head->value, head);
head = head->next;
}
return 0;
}
```
当你运行这个程序时,会打印出每个节点的值及其地址。注意,由于链表的动态性质,新插入的节点可能会改变现有节点的地址。这是因为链表元素的位置可以根据插入顺序而变化。
阅读全文
相关推荐
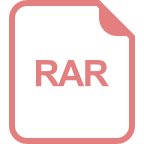
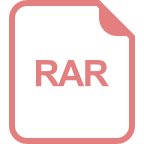
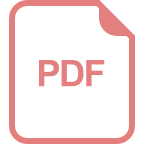

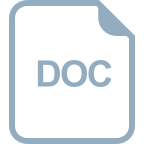
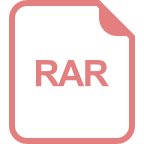
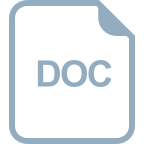
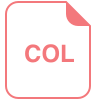


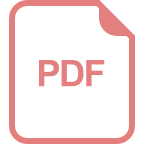
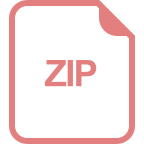
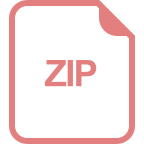
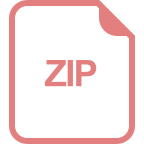
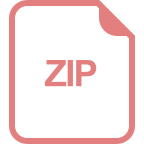
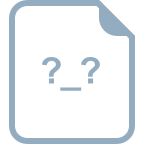
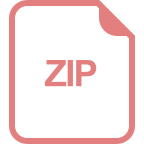
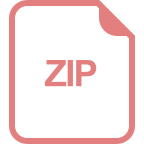