用java新建2个线程thread1, thread2。其中:thread1 采用 ThreadPoolExecutor ,thread2采用Executors工具类实现,第一个thread1线程实现功能 求和 1+2!+3!+4!+…+8!. 第二个thread2线程实现功能 找出前1000位数中所有的完数,完数如果一个数恰好等于它的真因子之和,则称该数为“完全数”,例如:6=3+2+1;
时间: 2024-01-03 16:04:19 浏览: 82
好的,下面是Java代码实现:
```java
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.*;
public class Main {
public static void main(String[] args) {
// 创建线程池
ThreadPoolExecutor threadPoolExecutor = new ThreadPoolExecutor(2, 2, 0L, TimeUnit.MILLISECONDS,
new LinkedBlockingQueue<Runnable>());
// 线程1求和 1+2!+3!+4!+…+8!
threadPoolExecutor.execute(() -> {
int sum = 0;
int fac = 1;
for (int i = 1; i <= 8; i++) {
fac *= i;
sum += fac;
}
System.out.println("1+2!+3!+4!+…+8! = " + sum);
});
// 线程2找出前1000位数中所有的完数
threadPoolExecutor.execute(() -> {
List<Integer> result = new ArrayList<>();
for (int i = 1; i <= 1000; i++) {
int sum = 0;
for (int j = 1; j < i; j++) {
if (i % j == 0) {
sum += j;
}
}
if (sum == i) {
result.add(i);
}
}
System.out.println("前1000位数中的完数:" + result);
});
// 关闭线程池
threadPoolExecutor.shutdown();
}
}
```
其中,线程1采用 ThreadPoolExecutor 实现,线程2采用 Executors 工具类实现。线程1求解1+2!+3!+4!+…+8!,线程2找出前1000位数中所有的完数。在这里,我使用了 List<Integer> 存储完数。最后,需要关闭线程池。
阅读全文
相关推荐



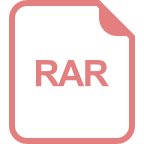

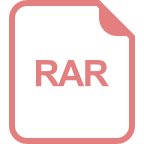
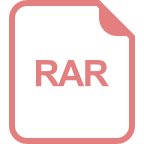










