JAVA中 xml字符串怎么转json
时间: 2023-02-21 09:26:15 浏览: 75
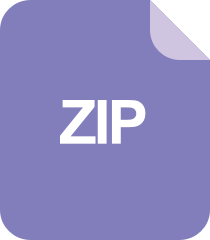
java 把xml转化为json
可以使用第三方库,比如 JSON-lib、Jackson 等来实现 XML 字符串到 JSON 字符串的转换。
具体的做法如下:
1. 先将 XML 字符串转换为 org.w3c.dom.Document 对象。
2. 使用 Jackson 的 XmlMapper 将 Document 对象映射为 JSON 对象。
3. 最后使用 Jackson 的 ObjectMapper 将 JSON 对象转换为 JSON 字符串。
代码示例:
```
import java.io.IOException;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import org.w3c.dom.Document;
import org.xml.sax.SAXException;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.dataformat.xml.XmlMapper;
import com.fasterxml.jackson.databind.ObjectMapper;
public class XmlToJson {
public static void main(String[] args) throws ParserConfigurationException, SAXException, IOException, JsonProcessingException {
String xmlString = "<foo>\n" +
" <bar>\n" +
" <type foobar=\"test\">test type</type>\n" +
" <value>test value</value>\n" +
" </bar>\n" +
" <bar>\n" +
" <type>test type2</type>\n" +
" <value>test value2</value>\n" +
" </bar>\n" +
"</foo>";
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
Document document = builder.parse(new InputSource(new StringReader(xmlString)));
XmlMapper xmlMapper = new XmlMapper();
JsonNode jsonNode = xmlMapper.readTree(document);
ObjectMapper objectMapper = new ObjectMapper();
String jsonString = objectMapper.writeValueAsString(jsonNode);
System.out.println(jsonString);
}
}
```
阅读全文
相关推荐



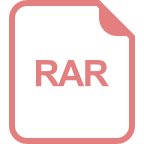


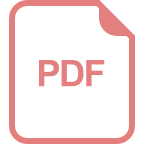
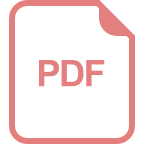
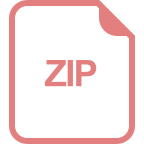
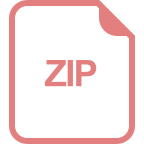
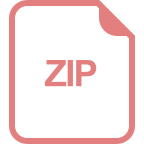
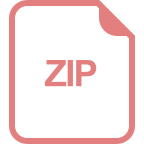
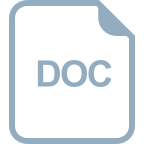

