Android studio 天气预报代码
时间: 2023-10-31 11:04:57 浏览: 200
这里是一个简单的 Android Studio 天气预报代码示例,使用了 OpenWeatherMap API:
1. 在 build.gradle 文件中添加以下依赖项:
```
implementation 'com.squareup.okhttp3:okhttp:3.10.0'
implementation 'com.google.code.gson:gson:2.8.2'
```
2. 创建一个 WeatherModel 类,用于存储天气数据:
```
public class WeatherModel {
private String cityName;
private String description;
private double temperature;
private String iconUrl;
public String getCityName() {
return cityName;
}
public void setCityName(String cityName) {
this.cityName = cityName;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public double getTemperature() {
return temperature;
}
public void setTemperature(double temperature) {
this.temperature = temperature;
}
public String getIconUrl() {
return iconUrl;
}
public void setIconUrl(String iconUrl) {
this.iconUrl = iconUrl;
}
}
```
3. 创建一个 WeatherService 类,用于获取天气数据:
```
public class WeatherService {
private static final String BASE_URL = "https://api.openweathermap.org/data/2.5/";
private static final String APP_ID = "YOUR_APP_ID_HERE";
private OkHttpClient okHttpClient;
private Gson gson;
public WeatherService() {
okHttpClient = new OkHttpClient();
gson = new Gson();
}
public WeatherModel getWeather(String cityName) throws IOException {
String url = BASE_URL + "weather?q=" + cityName + "&appid=" + APP_ID + "&units=metric";
Request request = new Request.Builder().url(url).build();
Response response = okHttpClient.newCall(request).execute();
String json = response.body().string();
WeatherModel model = gson.fromJson(json, WeatherModel.class);
model.setIconUrl(BASE_URL + "img/w/" + model.getIconUrl() + ".png");
return model;
}
}
```
4. 在 Activity 中使用 WeatherService 获取天气数据并显示:
```
public class MainActivity extends AppCompatActivity {
private static final String TAG = "MainActivity";
private EditText editText;
private TextView cityNameTextView;
private TextView temperatureTextView;
private TextView descriptionTextView;
private ImageView iconImageView;
private WeatherService weatherService;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
editText = findViewById(R.id.edit_text);
cityNameTextView = findViewById(R.id.city_name_text_view);
temperatureTextView = findViewById(R.id.temperature_text_view);
descriptionTextView = findViewById(R.id.description_text_view);
iconImageView = findViewById(R.id.icon_image_view);
weatherService = new WeatherService();
}
public void onGetWeatherClick(View view) {
String cityName = editText.getText().toString().trim();
if (TextUtils.isEmpty(cityName)) {
Toast.makeText(this, "Please enter a city name", Toast.LENGTH_SHORT).show();
return;
}
new Thread(() -> {
try {
WeatherModel model = weatherService.getWeather(cityName);
runOnUiThread(() -> {
cityNameTextView.setText(model.getCityName());
temperatureTextView.setText(getString(R.string.temperature, model.getTemperature()));
descriptionTextView.setText(model.getDescription());
Picasso.get().load(model.getIconUrl()).into(iconImageView);
});
} catch (IOException e) {
Log.e(TAG, "Failed to get weather", e);
runOnUiThread(() -> Toast.makeText(this, "Failed to get weather", Toast.LENGTH_SHORT).show());
}
}).start();
}
}
```
5. 在布局文件中添加以下组件:
```
<EditText
android:id="@+id/edit_text"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Enter City Name"
android:inputType="text"/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Get Weather"
android:onClick="onGetWeatherClick"/>
<TextView
android:id="@+id/city_name_text_view"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="24sp"/>
<TextView
android:id="@+id/temperature_text_view"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="48sp"/>
<TextView
android:id="@+id/description_text_view"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="24sp"/>
<ImageView
android:id="@+id/icon_image_view"
android:layout_width="100dp"
android:layout_height="100dp"/>
```
注意:在 WeatherService 中的 APP_ID 变量应该被替换为您在 OpenWeatherMap 上创建的 API 密钥。
阅读全文
相关推荐
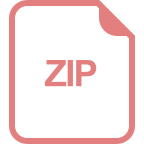


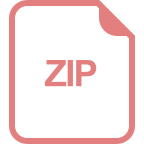
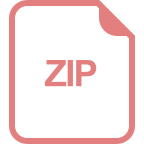











