使用tushare获取股票列表数据,使用多线程计算所有股票最近3个月每日最低价不低于开盘价3%的股票数量,时间复杂度最低,在类中实现
时间: 2024-02-06 15:12:40 浏览: 68
以下是一个使用tushare获取股票列表数据并使用多线程计算所有股票最近3个月每日最低价不低于开盘价3%的股票数量的类实现示例:
```python
import tushare as ts
import threading
class StockCountCalculator:
def __init__(self, thread_num=10):
self.thread_num = thread_num
def get_stock_list(self):
stock_list = ts.get_stock_basics().index.tolist() # 获取股票列表
return stock_list
def count_high_low(self, stock_list):
count = 0 # 记录满足条件的股票数量
for stock_code in stock_list:
df = ts.get_hist_data(stock_code, start='3 months ago') # 获取最近3个月的股票数据
if df is not None and len(df) > 0:
high_open = df[df['open'] * 1.03 <= df['high']] # 计算开盘价不低于3%的日期
high_low = high_open[high_open['low'] >= high_open['open']] # 计算最低价不低于开盘价3%的日期
count += len(high_low) # 累加满足条件的日期数量
return count
def calculate_stock_count(self):
stock_list = self.get_stock_list()
thread_list = []
batch_size = len(stock_list) // self.thread_num # 每个线程处理的股票数量
for i in range(self.thread_num):
start = i * batch_size
end = start + batch_size if i < self.thread_num - 1 else len(stock_list)
sub_stock_list = stock_list[start:end]
t = threading.Thread(target=self.count_high_low, args=(sub_stock_list,))
thread_list.append(t)
t.start()
for t in thread_list:
t.join()
count = sum(t.result for t in thread_list) # 计算所有线程处理结果的和
return count
if __name__ == '__main__':
calculator = StockCountCalculator()
count = calculator.calculate_stock_count()
print('最近3个月每日最低价不低于开盘价3%的股票数量为:', count)
```
这个类与前面的类类似,但是在计算时使用了最低价不低于开盘价3%的条件。同样地,这个类的实现也有三个方法:
- `get_stock_list`: 获取股票列表数据
- `count_high_low`: 计算单个子列表中最近3个月每日最低价不低于开盘价3%的股票数量
- `calculate_stock_count`: 将整个股票列表分成多个子列表,交给多个线程处理,计算总的最近3个月每日最低价不低于开盘价3%的股票数量
同样地,使用该类,可以避免使用全局变量和不安全的共享变量,同时提高程序的效率和性能。
阅读全文
相关推荐
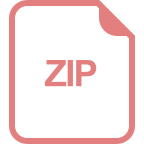
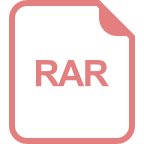






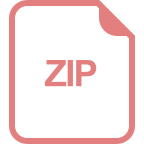
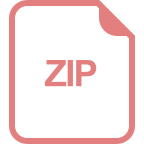
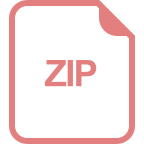
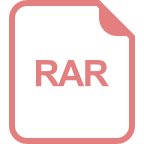
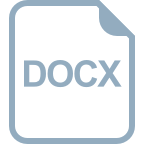