从tushare获取股票数据,多线程计算所有股票最近3个月最低价不低于开盘价3%的股票个数
时间: 2024-02-06 19:12:23 浏览: 78
好的,我理解了你的问题。首先,需要安装tushare库,并且获取tushare的token。然后,我们可以使用tushare的接口获取股票数据,计算每个股票最近3个月最低价不低于开盘价3%的情况,并统计符合条件的股票个数。为了提高效率,可以使用多线程进行计算。下面是一个简单的代码示例:
```python
import tushare as ts
import datetime
import threading
# 设置tushare token
ts.set_token('your_token')
# 获取股票列表
stock_list = ts.get_stock_basics().index.tolist()
# 定义计算函数
def calculate(stock):
# 获取最近3个月的股票数据
end_date = datetime.date.today().strftime('%Y-%m-%d')
start_date = (datetime.date.today() - datetime.timedelta(days=90)).strftime('%Y-%m-%d')
df = ts.get_hist_data(stock, start=start_date, end=end_date)
# 计算最低价和开盘价的比例
low_price = df['low'].min()
open_price = df.iloc[-1]['open']
ratio = low_price / open_price
# 判断是否符合条件
if ratio >= 0.97:
return True
else:
return False
# 定义多线程函数
def thread_function(stock_list, thread_num):
# 计算每个线程需要处理的股票数量
stock_num = len(stock_list)
thread_stock_num = stock_num // thread_num
# 定义线程列表
thread_list = []
# 启动线程
for i in range(thread_num):
start = i * thread_stock_num
end = (i + 1) * thread_stock_num if i != thread_num - 1 else stock_num
thread_list.append(threading.Thread(target=worker_function, args=(stock_list[start:end],)))
for t in thread_list:
t.start()
for t in thread_list:
t.join()
# 定义工作函数
def worker_function(stock_list):
# 统计符合条件的股票数量
count = 0
for stock in stock_list:
if calculate(stock):
count += 1
print(f'线程 {threading.current_thread().name} 处理了 {len(stock_list)} 只股票,符合条件的有 {count} 只。')
# 调用多线程函数
thread_function(stock_list, 4)
```
这个代码示例中,我们首先使用tushare库获取所有股票的代码列表,然后使用多线程进行计算。每个线程负责处理一部分股票,计算符合条件的股票个数。最后,将每个线程处理的结果进行累加,得到最终的结果。需要注意的是,由于tushare的接口有调用次数限制,如果股票数量太多,需要适当控制调用的频率以避免超出限制。
阅读全文
相关推荐
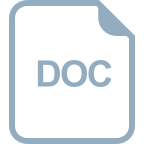
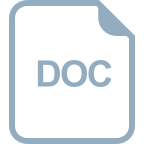
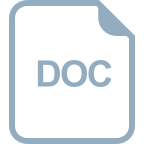






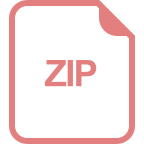
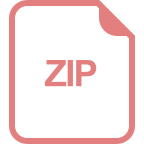
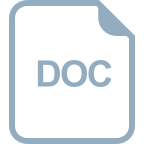
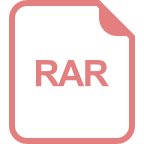
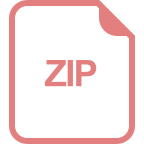
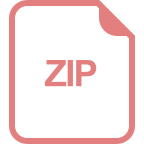
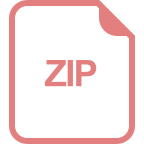
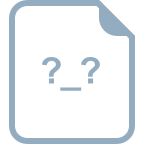