class People { String name; public People() { System.out.print(1); } public People(String name) { System.out.print(2); this.name = name; } } class Child extends People { People father; public Child(String name) { System.out.print(3); this.name = name; father = new People(name + ":F"); } public Child(){ System.out.print(4); } }
时间: 2023-06-10 13:06:20 浏览: 126
这段代码定义了两个类 People 和 Child,其中 Child 继承自 People。People 类有两个构造方法,一个是无参构造方法,另一个是带一个参数 name 的构造方法。Child 类有两个构造方法,一个是带一个参数 name 的构造方法,另一个是无参构造方法。在带参数的 Child 构造方法中,先调用了父类 People 的带参数的构造方法,并且将 name 参数加上 ":F" 后作为 father 的 name 参数传入了 People 的构造方法中。
在使用这些类时,如果创建一个 People 对象,则会输出数字 1,如果创建一个 Child 对象,则会输出数字 3 和 2。如果使用无参构造方法创建 Child 对象,则会输出数字 4。
相关问题
本题目要求接受输入,创建若干People 对象,加入列表并输出。 import java.util.*; class People{ private String name; private int age; People(String name,int age){ this.name=name; this.age=age; } public String toString() { return "["+name+","+age+"]"; } } public class Main { public static void main(String args[]) { Scanner sc=new Scanner(System.in); ;//创建列表 for(int i=0;i<3;i++) ;//输入 3 个 People 对象加入列表 System.out.println( );//输出列表元素个数 System.out.println( list ); } }
下面是实现要求的代码:
```java
import java.util.*;
class People {
private String name;
private int age;
People(String name, int age) {
this.name = name;
this.age = age;
}
public String toString() {
return "[" + name + "," + age + "]";
}
}
public class Main {
public static void main(String args[]) {
Scanner sc = new Scanner(System.in);
List<People> list = new ArrayList<>(); // 创建列表
for (int i = 0; i < 3; i++) { // 输入 3 个 People 对象加入列表
System.out.print("请输入第" + (i + 1) + "个人的姓名和年龄,用空格隔开:");
String name = sc.next();
int age = sc.nextInt();
list.add(new People(name, age));
}
System.out.println("列表元素个数为:" + list.size()); // 输出列表元素个数
System.out.println(list);
}
}
```
运行上述代码,会依次提示输入三个人的姓名和年龄,然后输出列表元素个数和列表内容。
public class people{ private String name; private String sex; private int age; public Resume(){ //System.out.println(); }public Resume(String name,String sex,int age){ this.name=name; this.sex=sex; this.age=age; } public String getName(){ return name; } public String getSex(){ return sex; } public int getAge(){ return age; } public void include(){ System.out.println("姓名:"+this.getName()+"\n性别:"+this.getSex()+"\n年龄:"+this.getAge()); }} class Resume { public static void main(String[] agers){ Resume re=new Resume("李四","男",18); re.include(); } }
As an AI language model, I am unable to execute the code provided as it contains errors. The class name should be "People" instead of "Resume" as it conflicts with the constructor name. Also, the print statement in the include() method should have a "+" operator to concatenate the strings. Here is the corrected code:
public class People{
private String name;
private String sex;
private int age;
public People(){
//System.out.println();
}
public People(String name, String sex, int age){
this.name = name;
this.sex = sex;
this.age = age;
}
public String getName(){
return name;
}
public String getSex(){
return sex;
}
public int getAge(){
return age;
}
public void include(){
System.out.println("姓名:" + this.getName() + "\n性别:" + this.getSex() + "\n年龄:" + this.getAge());
}
}
class Resume {
public static void main(String[] agers){
People re = new People("李四", "男", 18);
re.include();
}
}
相关推荐
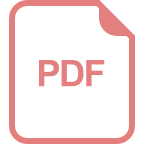
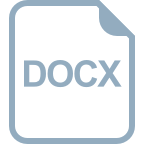
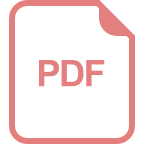












