StringUtil.isBlank函数的使用方法
时间: 2024-05-11 16:17:09 浏览: 7
StringUtil.isBlank函数是一个常用的字符串判断工具方法,用于判断一个字符串是否为空或者全是空格字符。它的使用方法如下:
```java
import org.apache.commons.lang3.StringUtils;
public class TestStringUtil {
public static void main(String[] args) {
String str1 = null;
String str2 = "";
String str3 = " ";
boolean isBlank1 = StringUtils.isBlank(str1); // true
boolean isBlank2 = StringUtils.isBlank(str2); // true
boolean isBlank3 = StringUtils.isBlank(str3); // true
System.out.println(isBlank1);
System.out.println(isBlank2);
System.out.println(isBlank3);
}
}
```
需要注意的是,使用该函数前需要引入`org.apache.commons.lang3.StringUtils`类。
相关问题
StringUtil.isBlank()
StringUtil.isBlank()是一个常见的字符串处理工具方法,通常用于检查字符串是否为空或仅包含空格。这个方法通常用于验证用户输入或处理文本数据时进行判空操作。
具体实现可能因不同的编程语言而异,但通常的功能是判断一个字符串是否为null、空字符串或只包含空格字符。如果满足这些条件,StringUtil.isBlank()方法将返回true,否则返回false。
以下是一个Java语言中的示例使用方式:
```java
import org.apache.commons.lang3.StringUtils;
public class Main {
public static void main(String[] args) {
String str1 = null;
String str2 = "";
String str3 = " ";
String str4 = "Hello World";
System.out.println(StringUtils.isBlank(str1)); // true
System.out.println(StringUtils.isBlank(str2)); // true
System.out.println(StringUtils.isBlank(str3)); // true
System.out.println(StringUtils.isBlank(str4)); // false
}
}
```
上述示例使用了Apache Commons Lang库中的StringUtils类来调用isBlank()方法。如果使用其他编程语言或其他字符串处理库,具体的调用方式可能会有所不同。
StringUtil.isBlank
StringUtils.isBlank是一个常用的字符串工具类方法,用于判断一个字符串是否为空或者只包含空格。[1]如果字符串为null、空字符串或者只包含空格,该方法会返回true;否则返回false。[1]相反的方法是StringUtils.isNotBlank,它用于判断一个字符串是否不为空且不只包含空格。[2]在示例代码中,StringUtils.isBlank("test")会返回false,因为字符串"test"既不是null也不是空字符串,也不只包含空格。[3]
相关推荐
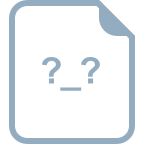
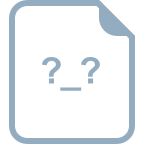












