写一个c++函数,输入为一个长方体的8个三维坐标点,把这个长方体在某个三维方向向量上拉长一倍
时间: 2023-06-25 18:07:44 浏览: 93
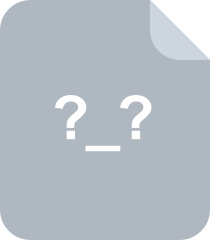
c++用函数写输入三边——判断三角形形状并计算面积周长

以下是一个简单的 C++ 函数,它实现了将长方体在某个三维方向向量上拉长一倍的操作:
```c++
#include <iostream>
#include <cmath>
using namespace std;
// 定义三维点结构体
struct point3d {
double x;
double y;
double z;
};
// 计算两个三维点之间的距离
double distance(point3d p1, point3d p2) {
double dx = p1.x - p2.x;
double dy = p1.y - p2.y;
double dz = p1.z - p2.z;
return sqrt(dx * dx + dy * dy + dz * dz);
}
// 将长方体在某个三维方向向量上拉长一倍
void elongate(point3d points[8], point3d dir) {
// 计算长方体的中心点
point3d center;
center.x = (points[0].x + points[6].x) / 2.0;
center.y = (points[0].y + points[6].y) / 2.0;
center.z = (points[0].z + points[6].z) / 2.0;
// 将每个点沿着指定的方向向量移动
for (int i = 0; i < 8; i++) {
double d = distance(points[i], center);
double scale = 2.0 * d / distance(dir, {0, 0, 0});
points[i].x += dir.x * scale;
points[i].y += dir.y * scale;
points[i].z += dir.z * scale;
}
}
int main() {
// 定义一个长方体的8个点
point3d points[8];
points[0] = {0, 0, 0};
points[1] = {1, 0, 0};
points[2] = {0, 1, 0};
points[3] = {1, 1, 0};
points[4] = {0, 0, 1};
points[5] = {1, 0, 1};
points[6] = {0, 1, 1};
points[7] = {1, 1, 1};
// 指定拉长的方向向量
point3d dir = {1, 1, 1};
// 执行拉长操作
elongate(points, dir);
// 输出拉长后的结果
for (int i = 0; i < 8; i++) {
cout << "(" << points[i].x << ", " << points[i].y << ", " << points[i].z << ")" << endl;
}
return 0;
}
```
在这个函数中,我们首先定义了一个三维点结构体,用于表示长方体的8个顶点。然后,我们实现了一个 `distance` 函数,用于计算两个三维点之间的距离。接着,我们定义了一个 `elongate` 函数,它接受一个长方体的8个顶点以及一个三维方向向量作为参数,并且将长方体沿着指定的方向向量拉长一倍。具体实现中,我们首先计算了长方体的中心点,然后对每个顶点进行移动,移动的距离是当前顶点到中心点的距离乘以2除以指定的方向向量的长度。最后,我们在 `main` 函数中定义了一个长方体的8个顶点,并指定了一个三维方向向量,然后调用了 `elongate` 函数,输出了拉长后的结果。
阅读全文
相关推荐
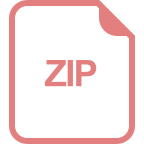

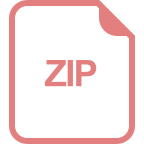
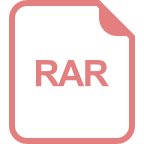
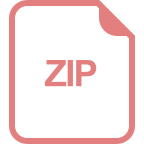
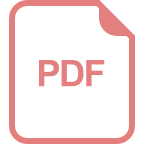
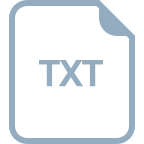
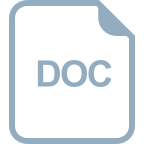
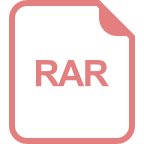
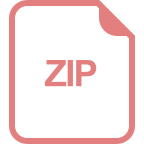
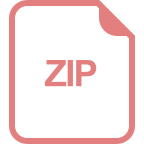
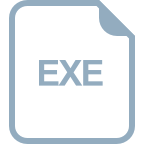
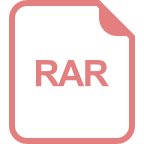
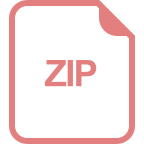
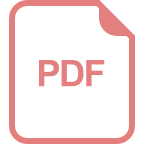