用c++解决问题:给定一个只包含小写字母的字符串,请你找到第一个仅出现一次的字符。如果没有,输出no
时间: 2024-10-17 12:03:48 浏览: 17
在C++中,解决这个问题可以采用哈希表的数据结构。我们可以遍历字符串,对于每个字符,检查它是否已经在哈希表中。如果不在,就将其添加到哈希表并设置计数为1;如果已经存在,说明这个字符不是第一次出现,更新其计数。最后再从哈希表中查找计数为1的字符即可。
以下是简单的C++代码实现:
```cpp
#include <unordered_map>
using namespace std;
char firstUniqChar(string s) {
unordered_map<char, int> charCount;
for (char c : s) {
if (charCount.find(c) == charCount.end()) { // 如果字符不存在
charCount[c] = 1; // 添加到哈希表,并计数为1
} else {
charCount[c]++; // 否则,计数加1
}
}
for (auto &entry : charCount) {
if (entry.second == 1) { // 找到计数为1的字符
return entry.first;
}
}
return 'no'; // 没有找到仅出现一次的字符,返回'n'
}
```
相关问题
用C++完成算法:给定一个只包含小写字母的字符串,请你找到第一个仅出现一次的字符。如果没有,输出no。
可以使用哈希表来解决这个问题。首先遍历一遍字符串,将每个字符出现的次数记录在哈希表中。然后再遍历一遍字符串,找到第一个出现次数为1的字符即可。
C 代码实现如下:
#include <stdio.h>
#include <string.h>
#define MAXN 100005
int cnt[26]; // 哈希表,记录每个字符出现的次数
int main()
{
char s[MAXN];
scanf("%s", s);
int n = strlen(s);
for (int i = ; i < n; i++) {
cnt[s[i] - 'a']++;
}
int ans = -1;
for (int i = ; i < n; i++) {
if (cnt[s[i] - 'a'] == 1) {
ans = i;
break;
}
}
if (ans == -1) {
printf("no\n");
} else {
printf("%c\n", s[ans]);
}
return ;
}
用c++编写:给定一个只包含小写字母的字符串,请你找到第一个仅出现一次的字符。如果没有,输出no
好的,我可以帮你用 C++ 编写。这里提供一种基于哈希表的解法,时间复杂度为 O(n)。
```c++
#include <iostream>
#include <unordered_map>
#include <string>
using namespace std;
char firstUniqChar(string s) {
unordered_map<char, int> count; // 定义哈希表
for (char c : s) {
count[c]++; // 统计每个字符出现的次数
}
for (char c : s) {
if (count[c] == 1) { // 找到第一个出现次数为1的字符
return c;
}
}
return 'n'; // 没有找到
}
int main() {
string s = "leetcode";
char c = firstUniqChar(s);
if (c != 'n') {
cout << "第一个仅出现一次的字符是:" << c << endl;
} else {
cout << "no" << endl;
}
return 0;
}
```
以上代码中,我们使用了 `unordered_map` 类型的哈希表来存储每个字符出现的次数。具体来说,我们遍历字符串 `s`,统计每个字符出现的次数,然后再次遍历 `s`,找到第一个出现次数为 1 的字符即可。如果没有找到,则返回 `n`,输出 `no`。
阅读全文
相关推荐
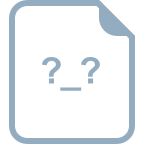
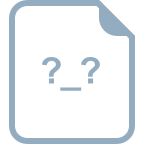
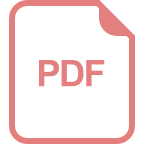













