通过v-for循环生成的div如何操控
时间: 2023-10-13 15:43:49 浏览: 47
通过v-for循环生成的div可以通过以下方法进行操控:
1. 使用v-bind指令绑定一个唯一的key属性到每个生成的div上,确保每个元素都有一个唯一的标识符。
```html
<div v-for="(item, index) in items" :key="index">{{ item }}</div>
```
2. 使用v-model指令来绑定每个生成的div上的数据,以实现双向数据绑定。
```html
<div v-for="(item, index) in items" :key="index">
<input type="text" v-model="item">
</div>
```
3. 通过在方法中操作数据来操控生成的div。你可以在Vue实例中定义一个方法,并在需要的时候调用它来修改相应的数据。
```html
<div v-for="(item, index) in items" :key="index">
<button @click="updateItem(index)">Update</button>
</div>
```
```javascript
methods: {
updateItem(index) {
this.items[index] = "New Value";
}
}
```
通过这些方法,你可以根据需要操控通过v-for循环生成的div元素。
相关问题
v-for循环生成el-button
您可以使用Vue.js中的v-for指令来循环生成el-button组件。下面是一个示例代码:
```html
<template>
<div>
<el-button v-for="button in buttons" :key="button.id">{{ button.text }}</el-button>
</div>
</template>
<script>
export default {
data() {
return {
buttons: [
{ id: 1, text: 'Button 1' },
{ id: 2, text: 'Button 2' },
{ id: 3, text: 'Button 3' }
]
};
}
};
</script>
```
在上面的代码中,我们使用v-for指令来循环遍历`buttons`数组,并为每个元素生成一个el-button组件。`:key`属性用于帮助Vue.js跟踪每个生成的组件,以提高性能。`button.text`用于显示按钮的文本内容。
您可以根据您的需求修改`buttons`数组中的数据来生成不同的按钮。
vue v-for 循环div div中包含一个按钮,点击按钮改变div 的背景颜色
好的,可以使用 Vue 的数据绑定和事件绑定来实现这个需求。你可以先使用 v-for 循环生成多个 div,然后每个 div 中包含一个按钮,绑定一个点击事件,在事件处理函数中改变该 div 的背景颜色。
具体代码如下:
```html
<template>
<div>
<div v-for="(item, index) in items" :key="index" :style="{ backgroundColor: item.color }">
<button @click="changeColor(index)">Change Color</button>
</div>
</div>
</template>
<script>
export default {
data() {
return {
items: [
{ color: 'red' },
{ color: 'blue' },
{ color: 'green' },
],
};
},
methods: {
changeColor(index) {
// 改变指定索引的 div 的背景颜色
this.items[index].color = 'yellow';
},
},
};
</script>
```
这里使用 v-for 循环生成了多个 div,每个 div 的背景颜色使用数据 items 中的 color 属性来设置。在每个 div 中还包含一个按钮,绑定了一个点击事件 changeColor,该事件接收一个参数 index,表示要改变的 div 的索引。在事件处理函数中,可以通过修改 items 数组中指定索引的元素的 color 属性来改变对应 div 的背景颜色。
相关推荐
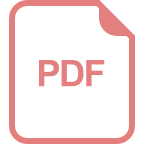
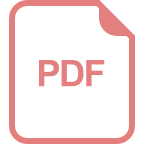












