如何使用C语言实现一个链表数据结构来管理学生通讯录?请结合具体示例代码说明。
时间: 2024-12-05 11:35:37 浏览: 26
在设计一个学生通讯录管理系统时,使用链表作为数据结构是一种非常合适的选择。链表允许我们在运行时动态地管理数据,非常适合通讯录这种需要频繁插入和删除数据的应用场景。下面我将结合示例代码,详细介绍如何使用C语言实现这样的系统。
参考资源链接:[学生通讯录管理系统设计与实现](https://wenku.csdn.net/doc/14045npx22?spm=1055.2569.3001.10343)
首先,我们定义一个学生信息的结构体(DataType),用来存储学生通讯录中的每条信息:
```c
typedef struct StudentInfo {
char studentID[20];
char name[50];
char gender[10];
char class[20];
char phone[20];
char address[100];
struct StudentInfo *next; // 指向下一个节点的指针
} DataType;
```
接下来,我们需要实现几个基本的操作函数,包括链表的创建、插入、删除和查找。以下是这些函数的示例代码:
```c
// 创建链表,初始化通讯录
DataType* LinkListCreateList(void) {
DataType *head = NULL, *p = NULL;
return head;
}
// 在链表中插入新的学生信息
void InsertNode(DataType *head, DataType *p) {
if (head == NULL) {
head = p;
p->next = NULL;
} else {
DataType *current = head;
while (current->next != NULL) {
current = current->next;
}
current->next = p;
p->next = NULL;
}
}
// 查找链表中的学生信息
DataType* ListFind(DataType *head, char *studentID) {
DataType *current = head;
while (current != NULL && strcmp(current->studentID, studentID) != 0) {
current = current->next;
}
return current;
}
// 删除链表中的学生信息
void DelNode(DataType **head, char *studentID) {
DataType *current = *head, *previous = NULL;
while (current != NULL && strcmp(current->studentID, studentID) != 0) {
previous = current;
current = current->next;
}
if (previous == NULL) {
*head = current->next;
} else {
previous->next = current->next;
}
free(current);
}
```
在实现这些函数之后,我们可以编写一个简单的主函数来测试它们:
```c
int main() {
DataType *head = LinkListCreateList();
DataType *newStudent = (DataType *)malloc(sizeof(DataType));
strcpy(newStudent->studentID,
参考资源链接:[学生通讯录管理系统设计与实现](https://wenku.csdn.net/doc/14045npx22?spm=1055.2569.3001.10343)
阅读全文
相关推荐
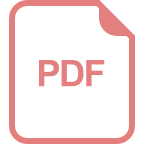
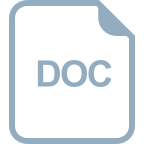
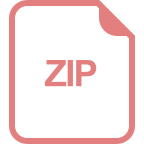
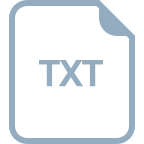
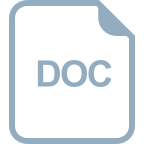
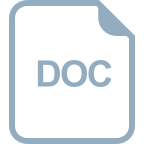
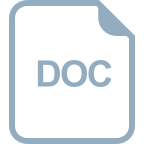
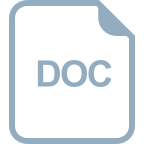
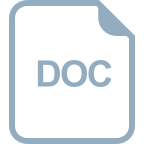








