数据结构:帮我用C语言制作一个学生通讯录管理系统
时间: 2024-12-11 07:40:07 浏览: 30
在C语言中创建一个简单的学生通讯录管理系统,我们可以使用链表数据结构来存储学生的姓名和联系方式。这里我会提供一个基本的框架和部分关键代码示例,实际应用中可能需要更完整的错误处理和用户界面。
首先,我们需要定义一个`Student`结构体:
```c
typedef struct {
char name[50];
char phone[20];
} Student;
```
接着,我们创建一个`List`结构体,用于链表操作:
```c
typedef struct List {
Student* head;
int size;
} List;
```
然后实现链表相关的函数,如创建、添加、删除和查找学生:
```c
// 创建一个新的链表
List* createList() {
List* list = (List*)malloc(sizeof(List));
list->head = NULL;
list->size = 0;
return list;
}
// 添加新学生到链表尾部
void addStudent(List* list, const char* name, const char* phone) {
Student* newNode = (Student*)malloc(sizeof(Student));
strcpy(newNode->name, name);
strcpy(newNode->phone, phone);
newNode->next = NULL;
if (list->head == NULL) {
list->head = newNode;
} else {
Student* temp = list->head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
list->size++;
}
// 删除指定名字的学生
void deleteStudent(List* list, const char* name) {
if (list->head == NULL) {
return;
}
if (strcmp(list->head->name, name) == 0) {
Student* temp = list->head;
list->head = list->head->next;
free(temp);
list->size--;
return;
}
Student* prev = list->head;
Student* curr = list->head->next;
while (curr != NULL && strcmp(curr->name, name) != 0) {
prev = curr;
curr = curr->next;
}
if (curr != NULL) {
prev->next = curr->next;
free(curr);
list->size--;
}
}
// 查找并打印所有学生信息
void printStudents(const List* list) {
if (list->head == NULL) {
printf("No students in the list.\n");
return;
}
Student* temp = list->head;
while (temp != NULL) {
printf("Name: %s, Phone: %s\n", temp->name, temp->phone);
temp = temp->next;
}
}
```
阅读全文
相关推荐
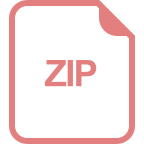
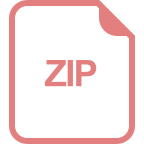
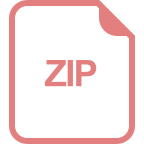
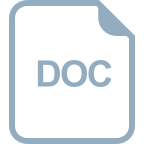
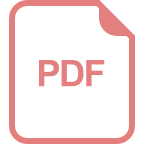
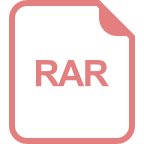
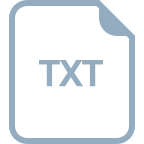
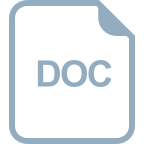
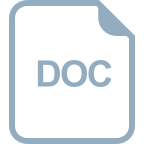
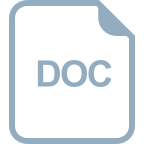
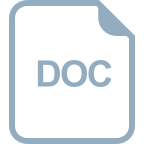
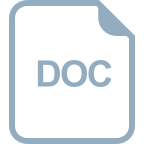
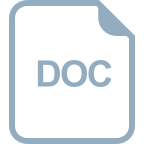
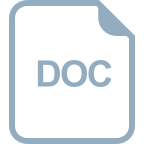
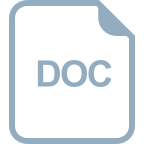


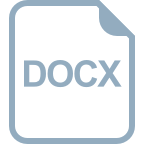