thymeleaf ajax
时间: 2023-10-31 16:57:45 浏览: 140
Thymeleaf is a server-side Java template engine used for web development. It allows developers to create dynamic web pages by blending HTML with server-side code. Thymeleaf supports AJAX (Asynchronous JavaScript and XML) requests, which allows you to update parts of a web page without reloading the entire page.
To use Thymeleaf with AJAX, you can follow these steps:
1. Include Thymeleaf dependencies in your project.
2. Create a Thymeleaf template that contains the HTML structure you want to update dynamically.
3. Use Thymeleaf attributes to bind data from the server-side to your HTML elements.
4. Create an AJAX request to send data to the server and retrieve the updated content.
5. Handle the AJAX response and update the specific HTML elements with the new content using JavaScript.
Here's an example of how you can use Thymeleaf with AJAX:
1. Thymeleaf template (example.html):
```html
<div id="messageContainer">
<p th:text="${message}"></p>
</div>
<button onclick="sendMessage()">Send Message</button>
<script>
function sendMessage() {
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState === 4 && this.status === 200) {
document.getElementById("messageContainer").innerHTML = this.responseText;
}
};
xhttp.open("GET", "/sendMessage", true);
xhttp.send();
}
</script>
```
2. Controller class:
```java
@Controller
public class MessageController {
@GetMapping("/sendMessage")
public String sendMessage(Model model) {
// Perform some logic or retrieve data
String message = "Hello, world!";
model.addAttribute("message", message);
return "messageFragment :: message";
}
}
```
In the above example, when the "Send Message" button is clicked, an AJAX request is sent to the server's "/sendMessage" endpoint. The server-side logic performs some operations or retrieves data and returns the updated message. The Thymeleaf template then updates the "messageContainer" div with the new content without reloading the entire page.
Note that the Thymeleaf fragment "messageFragment :: message" is returned from the "/sendMessage" endpoint. This allows only the "message" fragment of the template to be updated instead of the entire page.
阅读全文
相关推荐
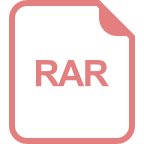
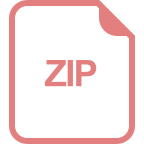














