编写程序利用直方图均衡方法对图像进行增强处理,要求不用opencv函数实现
时间: 2024-12-24 14:38:07 浏览: 11
当然可以,不使用 OpenCV 函数的情况下,我们可以手动实现直方图均衡化。以下是一个基本的 Python 示例,使用了 NumPy 和一些基本操作:
```python
import numpy as np
def histogram(image):
flattened_img = image.flatten() # 展平图像数组
counts, _ = np.unique(flattened_img, return_counts=True) # 统计每个像素值的数量
return counts
def cumulative_distribution(counts):
total_pixels = counts.sum()
return counts.cumsum() / total_pixels # 计算累计概率分布
def rescale_image(image, cumulative_dist):
flattened_img = image.flatten()
new_values = np.searchsorted(cumulative_dist, flattened_img) # 查找新像素值对应的累积分布位置
reshaped_img = new_values.reshape(image.shape) # 重塑回原图像形状
return reshaped_img
def histogram_equation(image):
counts = histogram(image)
cumulative_dist = cumulative_distribution(counts)
enhanced_image = rescale_image(image, cumulative_dist)
return enhanced_image
# 示例图像
image = np.random.randint(0, 256, size=(200, 200), dtype=np.uint8) # 假设这是你的图像数据
enhanced_image = histogram_equation(image)
```
这段代码首先计算原始图像的直方图,然后计算累计分布,最后通过搜索排序将像素值映射到新的概率分布上,实现了直方图均衡。
阅读全文
相关推荐
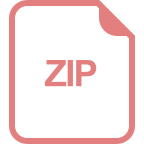
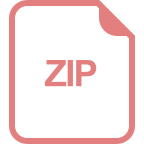
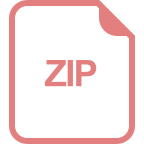



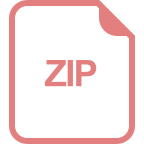
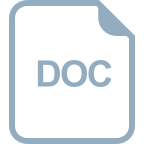
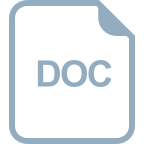
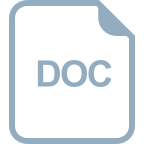
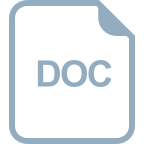
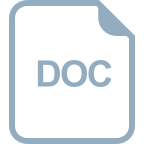
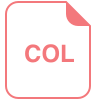
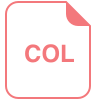
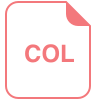
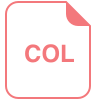


