编写程序利用直方图均衡化方法对图像增强,要求不用opencv函数实现
时间: 2024-12-24 10:38:04 浏览: 7
直方图均衡化是一种常用的图像增强技术,通过调整像素值分布来提高图像对比度,使得灰度图像更均匀地分布在0到255的范围内。如果你想要不使用OpenCV库直接编写程序实现直方图均衡化,可以按照以下步骤:
1. **读取图像**:
首先,你需要从文件或内存中读取图像数据。这通常涉及到将图像转换为一维数组或矩阵。
```python
def read_image(file_path):
with open(file_path, 'rb') as f:
image_data = f.read()
# 将二进制数据转化为灰度图像
width, height = ... # 获取图像尺寸
pixels = [image_data[i * width : (i+1) * width] for i in range(height)]
return pixels
```
2. **计算原图像的直方图**:
对每个像素灰度值统计其频率,形成原始的直方图。
```python
def histogram(pixels):
hist = [0] * 256
for row in pixels:
for pixel in row:
hist[pixel] += 1
return hist
```
3. **构建累积分布函数(CDF)**:
根据直方图计算每个灰度值对应的累积概率。
```python
def cumulative_distribution(hist):
cdf = [0]
for count in hist:
cdf.append(cdf[-1] + count / sum(hist))
return cdf
```
4. **应用累积分布函数**:
使用CDF将原图像中的每个像素映射到新的灰度值范围。
```python
def apply_cdf(image_pixels, cdf):
processed_pixels = []
for row in image_pixels:
new_row = [int((cdf[pixel_value] * 255).round()) for pixel_value in row]
processed_pixels.append(new_row)
return processed_pixels
```
5. **显示或保存处理后的图像**:
最后,你可以把处理后的像素数据转换回图像格式并展示或保存。
```python
def display_or_save_image(processed_pixels, file_path):
new_image = ... # 将像素数据重构为适合显示或保存的格式
save_image(new_image, file_path)
```
请注意,上述代码简化了实现过程,实际操作可能会涉及更多的边缘情况处理和性能优化。此外,由于篇幅限制,这里没有提供完整的错误处理和细节实现。你可以在网上找到更多关于图像处理的教程来完善这部分内容。
阅读全文
相关推荐
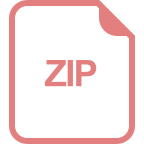
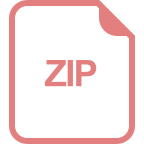
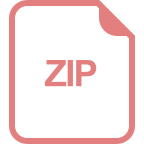


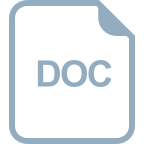

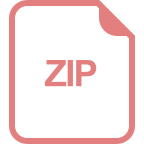
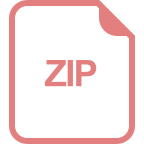
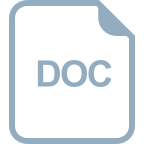
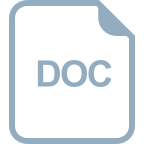
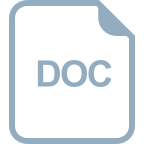
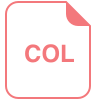
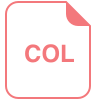
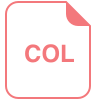
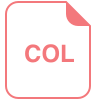


