Selenium模拟鼠标滚轮
时间: 2024-05-09 20:12:19 浏览: 14
Selenium可以使用ActionChains类来模拟用户的行为,其中包括鼠标滚轮。以下是模拟鼠标滚轮向下滚动1000像素的方法[^1]:
```python
from selenium import webdriver
from selenium.webdriver.common.action_chains import ActionChains
# 初始化 WebDriver
driver = webdriver.Chrome()
# 打开网页
driver.get("https://www.example.com")
# 执行鼠标滚轮操作
actions = ActionChains(driver)
actions.move_by_offset(0, 1000).perform() # 向下滚动1000像素
```
上面的代码中,我们使用了Chrome浏览器,并打开了一个示例网页。然后,使用ActionChains类创建一个动作链,调用move_by_offset方法并向下滚动1000像素。最后,调用perform方法执行此操作。
如果需要向上滚动,则需要将像素值改为负数,例如向上滚动1000像素,可以使用以下代码:
```python
actions.move_by_offset(0, -1000).perform() # 向上滚动1000像素
```
相关问题
selenium模拟鼠标滚轮
要模拟鼠标滚轮操作,可以使用Selenium中的`ActionChains`类。以下是一个示例代码,演示如何在Python中使用Selenium模拟鼠标滚轮向下滚动:
```python
from selenium.webdriver import ActionChains
# 假设你已经初始化了一个WebDriver实例,名为driver
# 定位到需要滚动的元素
element = driver.find_element_by_xpath("your_xpath_here")
# 创建一个ActionChains对象
actions = ActionChains(driver)
# 模拟鼠标滚轮向下滚动
actions.move_to_element(element).perform()
actions.move_to_element(element).send_keys(Keys.PAGE_DOWN).perform()
```
这个代码片段假设你要滚动的元素已经被定位到,并且你已经初始化了一个WebDriver实例,名为`driver`。你可以根据你的实际需要修改代码来实现你想要的操作。
selenium模拟鼠标滚轮滚动
使用ActionChains模拟鼠标滚轮滚动:
```python
from selenium.webdriver.common.action_chains import ActionChains
# 滚动到页面底部
def scroll_to_bottom(driver):
actions = ActionChains(driver)
actions.move_to_element(driver.find_element_by_tag_name('body'))
actions.click_and_hold()
actions.move_by_offset(0, 1000)
actions.release()
actions.perform()
```
其中,`move_by_offset()`方法可以控制滚动的距离,正数表示往下滚动,负数表示往上滚动。可以根据需要调整滚动距离。
相关推荐
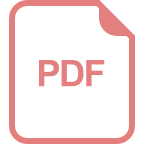












