vue3增删改查加本地存储
时间: 2024-09-20 15:01:25 浏览: 58
Vue3中的Vuex是一个状态管理模式,特别适合于管理全局组件之间的共享状态。当涉及到数据的增删改查操作时,我们可以结合Vuex使用:
1. **增**: 新建一个mutation(改变状态的动作)用于添加新的数据项。例如:
```javascript
mutations: {
ADD_ITEM(state, item) {
state.items.push(item);
}
}
```
然后在组件中通过dispatch触发这个mutation。
2. **删**: 另一个mutation处理删除操作,通常会接受一个id作为参数:
```javascript
mutations: {
DELETE_ITEM(state, id) {
state.items = state.items.filter(item => item.id !== id);
}
}
```
3. **改**: 同样创建一个mutation更新特定的数据:
```javascript
mutations: {
UPDATE_ITEM(state, updatedItem) {
const index = state.items.findIndex(item => item.id === updatedItem.id);
if (index !== -1) {
state.items[index] = updatedItem;
}
}
}
```
4. **查**: 数据获取通常通过actions(异步操作),如axios请求API:
```javascript
actions: {
fetchItems({ commit }) {
axios.get('/api/items').then(response => {
commit('SET_ITEMS', response.data);
});
}
}
```
最后,在组件中使用`mapActions`映射这个action到store。
至于本地存储,Vue提供了一些库如vue-local-storage、quasar-framework等,可以将Vuex的状态持久化。例如,保存数据前:
```javascript
beforeDestroy() {
localStorage.setItem('items', JSON.stringify(this.$store.state.items));
}
```
恢复时:
```javascript
created() {
try {
const storedItems = JSON.parse(localStorage.getItem('items'));
this.$store.commit('SET_ITEMS', storedItems);
} catch (error) {}
}
```
阅读全文
相关推荐
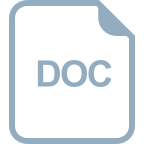
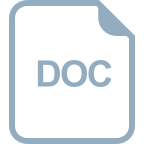
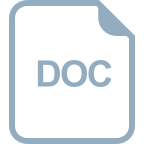

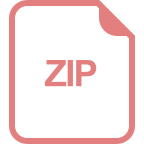
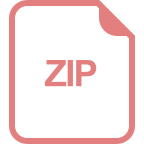
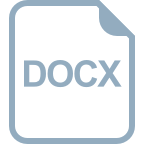




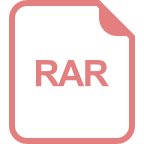
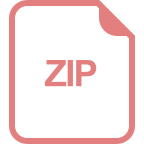
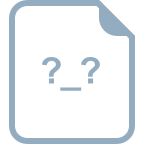
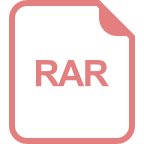
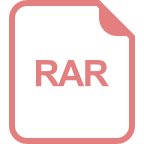
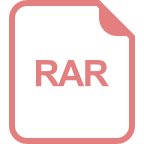
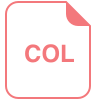
