用MASM编写一段32位汇编语言程序,要求:已知字符串“ABCDEFGH”位于数据段开始的区域中,编程序将该字符串转换成小写后,存于其后。不用函数
时间: 2024-12-18 18:23:14 浏览: 12
在MASM中,为了将给定的字符串从大写转换为小写,我们需要遍历整个字符串并将每个大写字母替换为其相应的小写字母。由于ASCII码表中小写字母的值比大写字母低,我们可以利用这一点来进行转换。这里有一个基本的示例:
```assembly
section .data
input_string db 'ABCDEFGH', 0 ; 输入的大写字符串
output_buffer times 8 db 0 ; 存放结果的小写字符串
section .text
global _start
_start:
; 初始化指针
lea ebx, [input_string] ; ebx指向输入字符串
lea edi, [output_buffer] ; edi指向输出缓冲区
convert_loop:
; 检查是否到达字符串结束
cmp byte [ebx], 0
je end_convert
; 将大写字母转换为小写
cmp byte [ebx], 'A' ; 如果是大写字母
jl skip_conversion ; 跳过非大写字母部分
cmp byte [ebx], 'Z'
jg skip_conversion ; 同理,跳过非大写字母部分
add byte [ebx], 32 ; ASCII码减去32得到对应的小写字母
skip_conversion:
mov [edi], byte [ebx] ; 将转换后的字符存储到输出缓冲区
inc ebx ; 移动到下一个字符
inc edi ; 移动到下一个位置
jmp convert_loop ; 继续下一次循环
end_convert:
; 设置终止符
mov byte [edi], 0
; 打印转换后的字符串
call print_output_buffer
; 结束程序
mov eax, 1 ; sys_exit syscall number
xor ebx, ebx ; exit code 0
int 0x80 ; call kernel
print_output_buffer:
push ebx ; save ebx for use in interrupt
push edi ; pass output buffer to WriteConsoleA
invoke WriteConsoleA, hStdOut, edi, edi+1, eax, NULL ; 输出缓冲区
add esp, 8 ; clean up the stack
pop ebx ; restore ebx from interrupt
ret ; return from interrupt
hStdOut equ -11 ; Standard Output handle
section .bss
hStdOut resd 1 ; reserve space for standard output handle
```
这个程序会直接修改`output_buffer`中的内容,并将其作为小写字符串输出。
阅读全文
相关推荐
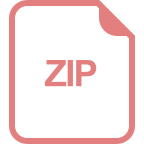
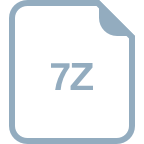

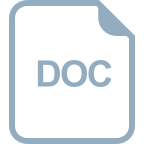
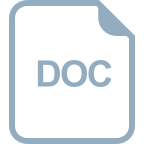
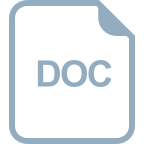
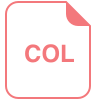

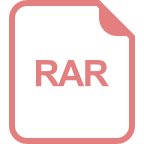
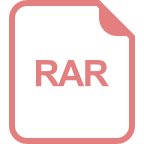
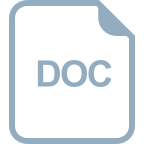
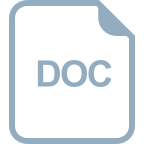
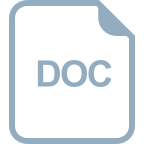
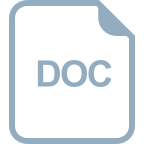
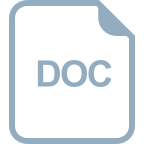
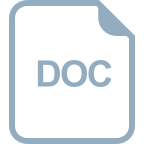


