Android实现高斯模糊背景对话框,Android实现毛玻璃效果的对话框
时间: 2023-08-05 14:41:20 浏览: 79
要实现高斯模糊背景的对话框,可以使用 Android 的 DialogFragment 和 RenderScript 实现。以下是大致的步骤:
1. 在布局文件中定义对话框的 UI,注意要为对话框添加一个半透明的背景。
2. 创建一个继承自 DialogFragment 的类,并在其中实现 onCreateDialog() 方法。
3. 在 onCreateDialog() 方法中,使用 RenderScript 创建一个高斯模糊的 Bitmap,并将其设置为对话框背景。
4. 在对话框显示时,使用 DialogFragment.show() 方法显示对话框。
以下是示例代码:
```java
public class BlurDialogFragment extends DialogFragment {
@Override
public Dialog onCreateDialog(Bundle savedInstanceState) {
// 创建一个对话框
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
LayoutInflater inflater = getActivity().getLayoutInflater();
View view = inflater.inflate(R.layout.dialog_layout, null);
builder.setView(view);
// 创建一个 RenderScript 对象
RenderScript rs = RenderScript.create(getActivity());
// 加载图片资源
Bitmap image = BitmapFactory.decodeResource(getResources(), R.drawable.background);
// 创建一个高斯模糊的 Bitmap
Bitmap blurredBitmap = image.copy(Bitmap.Config.ARGB_8888, true);
Allocation input = Allocation.createFromBitmap(rs, image);
Allocation output = Allocation.createFromBitmap(rs, blurredBitmap);
ScriptIntrinsicBlur script = ScriptIntrinsicBlur.create(rs, Element.U8_4(rs));
script.setInput(input);
script.setRadius(25.f);
script.forEach(output);
output.copyTo(blurredBitmap);
// 将高斯模糊的 Bitmap 设置为对话框背景
view.setBackground(new BitmapDrawable(getResources(), blurredBitmap));
return builder.create();
}
}
```
要实现毛玻璃效果的对话框,可以使用 Android 的 DialogFragment 和自定义控件实现。以下是大致的步骤:
1. 在布局文件中定义对话框的 UI,包括一个用于显示毛玻璃效果的自定义控件。
2. 创建一个继承自 DialogFragment 的类,并在其中实现 onCreateDialog() 方法。
3. 在 onCreateDialog() 方法中,创建一个自定义控件的实例,并将其添加到对话框的 UI 中。
4. 在对话框显示时,使用 DialogFragment.show() 方法显示对话框。
以下是示例代码:
```java
public class GlassDialogFragment extends DialogFragment {
@Override
public Dialog onCreateDialog(Bundle savedInstanceState) {
// 创建一个对话框
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
LayoutInflater inflater = getActivity().getLayoutInflater();
View view = inflater.inflate(R.layout.dialog_layout, null);
builder.setView(view);
// 创建一个自定义控件
GlassView glassView = new GlassView(getActivity());
ViewGroup.LayoutParams layoutParams = new ViewGroup.LayoutParams(
ViewGroup.LayoutParams.MATCH_PARENT,
ViewGroup.LayoutParams.MATCH_PARENT);
glassView.setLayoutParams(layoutParams);
// 将自定义控件添加到对话框的 UI 中
FrameLayout frameLayout = view.findViewById(R.id.frame_layout);
frameLayout.addView(glassView);
return builder.create();
}
}
```
其中 GlassView 是一个自定义控件,用于显示毛玻璃效果。以下是示例代码:
```java
public class GlassView extends View {
private Paint paint = new Paint();
private Bitmap bitmap;
public GlassView(Context context) {
super(context);
init();
}
public GlassView(Context context, AttributeSet attrs) {
super(context, attrs);
init();
}
public GlassView(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
init();
}
private void init() {
// 加载图片资源
bitmap = BitmapFactory.decodeResource(getResources(), R.drawable.background);
// 设置画笔透明度
paint.setAlpha(100);
}
@Override
protected void onDraw(Canvas canvas) {
// 创建一个缩小的 Bitmap,用于实现毛玻璃效果
Bitmap scaledBitmap = Bitmap.createScaledBitmap(bitmap, bitmap.getWidth() / 10, bitmap.getHeight() / 10, false);
// 将缩小的 Bitmap 放大,实现模糊效果
Bitmap blurredBitmap = Bitmap.createScaledBitmap(scaledBitmap, getWidth(), getHeight(), false);
// 绘制模糊的 Bitmap
canvas.drawBitmap(blurredBitmap, 0, 0, paint);
}
}
```
注意:以上代码只是示例代码,实际上还需要根据具体情况进行调整和优化。
相关推荐
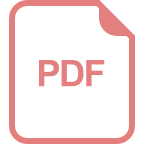
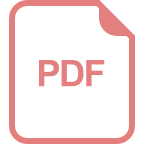














