python 画坐标轴
时间: 2023-09-09 11:12:17 浏览: 154
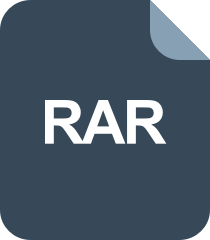
自动绘制坐标轴的工具

要在 Python 中画坐标轴,你可以使用 matplotlib 库。下面是一个示例代码,它将创建一个简单的坐标轴图形:
```python
import matplotlib.pyplot as plt
# 创建数据
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
# 创建图形
plt.plot(x, y)
# 添加标题和标签
plt.title("Plot of x and y")
plt.xlabel("x-axis")
plt.ylabel("y-axis")
# 显示图形
plt.show()
```
这段代码将生成一个简单的图形,其中 x 轴表示 `x` 列表的值,y 轴表示 `y` 列表的值。你可以根据需要修改数据和图形属性。记得在运行代码之前确保已经安装了 matplotlib 库。
阅读全文
相关推荐














