std::bind 函数使用
时间: 2023-07-23 17:23:57 浏览: 64
std::bind 是 C++11 中的一个函数模板,用于将一个函数对象或成员函数与其参数绑定,生成一个新的函数对象。
使用 std::bind 可以方便的实现函数的柯里化(Currying)操作,即将一个多参数的函数转化为一个接受少量参数的函数序列,每次调用返回一个新的函数对象,直到所有参数都被传递完毕。
std::bind 的基本语法如下:
```c++
#include <functional>
auto new_func = std::bind(func, arg1, arg2, ...);
```
其中,func 是需要绑定的函数对象或成员函数,arg1, arg2, ... 是需要绑定的参数。
例如,如果有一个函数 foo,接受两个 int 类型的参数,可以使用 std::bind 将其中一个参数绑定,生成一个新的函数对象:
```c++
#include <functional>
#include <iostream>
void foo(int a, int b) {
std::cout << a << " " << b << std::endl;
}
int main() {
auto new_func = std::bind(foo, 1, std::placeholders::_1);
new_func(2); // 输出:1 2
return 0;
}
```
在上面的例子中,使用 `std::placeholders::_1` 表示绑定第二个参数,生成一个新的函数对象 new_func,调用 new_func(2),实际上就相当于调用 foo(1, 2)。
另外,std::bind 还支持占位符的使用,占位符表示某个参数需要在调用时再传入,例如:
```c++
#include <functional>
#include <iostream>
void foo(int a, int b, int c) {
std::cout << a << " " << b << " " << c << std::endl;
}
int main() {
auto new_func = std::bind(foo, std::placeholders::_3, std::placeholders::_1, std::placeholders::_2);
new_func(1, 2, 3); // 输出:3 1 2
return 0;
}
```
在上面的例子中,使用 `std::placeholders::_1`、`std::placeholders::_2`、`std::placeholders::_3` 表示绑定第一个、第二个、第三个参数,生成一个新的函数对象 new_func,调用 new_func(1, 2, 3),实际上就相当于调用 foo(3, 1, 2)。
相关推荐
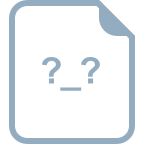
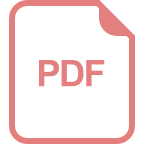
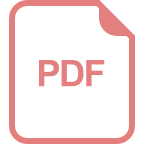
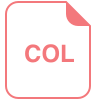













