C++ 从utf8文件中读入中文乱码怎么解决?
时间: 2024-03-14 08:47:10 浏览: 26
在C++中从UTF-8编码的文件中读取中文内容时,需要注意文件的编码格式和读取方式。以下是一些可能有帮助的方法:
1. 打开文件时指定编码格式
在打开文件时,需要将文件流的编码格式设置为UTF-8,以便正确地读取中文字符。可以使用`std::wifstream`类代替`std::ifstream`,并在打开文件时使用`std::locale("en_US.UTF-8")`指定编码格式。
```c++
#include <iostream>
#include <fstream>
#include <locale>
#include <codecvt>
int main() {
std::wifstream infile("filename.txt");
infile.imbue(std::locale("en_US.UTF-8"));
std::wstring line;
while (std::getline(infile, line)) {
std::wcout << line << std::endl;
}
infile.close();
return 0;
}
```
2. 使用`std::wstring_convert`进行编码转换
可以使用`std::wstring_convert`类将UTF-8编码的字符串转换为`std::wstring`类型。需要包含头文件`<codecvt>`和`<locale>`。
```c++
#include <iostream>
#include <fstream>
#include <locale>
#include <codecvt>
int main() {
std::ifstream infile("filename.txt");
infile.imbue(std::locale("en_US.UTF-8"));
std::string line;
std::wstring_convert<std::codecvt_utf8<wchar_t>> converter;
while (std::getline(infile, line)) {
std::wstring wline = converter.from_bytes(line);
std::wcout << wline << std::endl;
}
infile.close();
return 0;
}
```
以上两种方法都可以正确地读取UTF-8编码的文件中的中文字符,您可以根据自己的实际情况选择其中的一种方法。
相关推荐
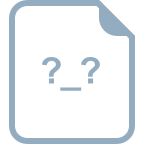
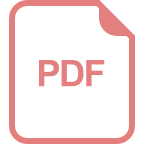
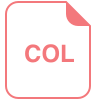
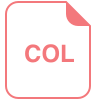
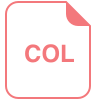
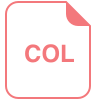
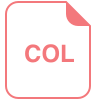









