If the user types something which is not an integer, then the nextInt method of the scanner will throw an InputMismatchException. In that case the code of your readPosInt method must catch the exception, use System.out.println to print the error message "You must type an integer!" to the user (use System.out.println for this, not System.err.println, otherwise you might hit a bug in Eclipse...), use the scanner’s nextLine method to read (and ignore) the wrong input typed by the user of the program (if you do not do this, the wrong input typed by the user will remain in the input stream, and the next time you call the nextInt method again, you will get an InputMismatchException again!), and then do the whole thing again (including printing again the string argument of the readPosInt method) to try to read an integer again (hint: put the whole code of the method inside a while loop).java代码
时间: 2024-01-25 17:02:47 浏览: 32
Sure, I understand your question. Here's a possible implementation of the `readPosInt` method in Java that handles the scenario you described:
```java
import java.util.InputMismatchException;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
int n = readPosInt("Please enter a positive integer: ");
System.out.println("You entered: " + n);
}
public static int readPosInt(String prompt) {
Scanner scanner = new Scanner(System.in);
int n = 0;
boolean success = false;
while (!success) {
System.out.print(prompt);
try {
n = scanner.nextInt();
if (n > 0) {
success = true;
} else {
System.out.println("You must type a positive integer!");
}
} catch (InputMismatchException e) {
System.out.println("You must type an integer!");
scanner.nextLine(); // consume wrong input
}
}
return n;
}
}
```
In this implementation, we use a `while` loop to keep trying to read an integer until we succeed. Inside the loop, we print the prompt and attempt to read an integer using `scanner.nextInt()`. If this call throws an `InputMismatchException`, we catch it and print the error message "You must type an integer!" to the user using `System.out.println()`. We also use `scanner.nextLine()` to consume the wrong input typed by the user, so that the input stream is properly cleared and the next call to `scanner.nextInt()` doesn't fail again.
If the input is an integer, we check if it's positive and set the `success` flag to `true` if it is. If the input is not positive, we print the error message "You must type a positive integer!" to the user using `System.out.println()`.
Finally, if we succeed in reading a positive integer, we return it from the method.
相关推荐
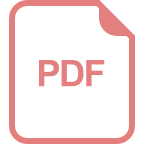
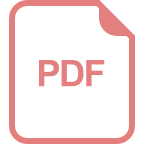
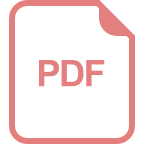















