调用random标准库,随机生成6位验证码,包含大小写,数字及字符。调用datetime和time标准库,每隔5秒显示一个随机验证码,并显示每个验证码及其产生时间
时间: 2024-12-14 07:14:21 浏览: 4
在Python中,你可以使用`random`库来生成随机验证码,`datetime`和`time`库来获取当前时间和间隔操作。下面是一个简单的示例:
```python
import random
import string
from datetime import datetime, timedelta
import time
# 定义生成随机验证码的函数
def generate_code(length=6):
characters = string.ascii_letters + string.digits + string.punctuation
return ''.join(random.choice(characters) for _ in range(length))
# 每隔5秒生成并打印验证码及其产生时间
while True:
code = generate_code()
current_time = datetime.now().strftime("%Y-%m-%d %H:%M:%S")
print(f"验证码: {code}")
print(f"产生时间: {current_time}")
# 等待5秒钟
time.sleep(5)
# 为了不影响下一轮循环,这里清除上一轮输出
# 在实际应用中,你可能希望保留历史记录,所以这一步可以根据需求修改
input("按回车继续...")
相关问题
常用标准库清单如下 sys os math random pikle subprocess queue StringIO logging json time datetime re csv threading asyncio 请用python实例代码
当然,我可以为您提供一些使用常用标准库的 Python 示例代码。以下是一个简单的例子:
```python
import sys
import os
import math
import random
import pickle
import subprocess
import queue
from io import StringIO
import logging
import json
import time
import datetime
import re
import csv
import threading
import asyncio
# 示例:使用random库生成一个随机数
random_number = random.randint(1, 100)
print("随机数:", random_number)
# 示例:使用os库获取当前工作目录
current_dir = os.getcwd()
print("当前工作目录:", current_dir)
# 示例:使用datetime库获取当前时间
current_time = datetime.datetime.now()
print("当前时间:", current_time)
# 示例:使用re库进行正则表达式匹配
pattern = r'\d+'
text = 'Hello 123 World'
matches = re.findall(pattern, text)
print("匹配结果:", matches)
# 示例:使用json库将字典对象转换为JSON字符串
data = {'name': 'John', 'age': 30, 'city': 'New York'}
json_str = json.dumps(data)
print("JSON字符串:", json_str)
# 示例:使用threading库创建并启动一个线程
def print_message(message):
print("线程消息:", message)
thread = threading.Thread(target=print_message, args=("Hello from thread",))
thread.start()
thread.join()
# 示例:使用asyncio库创建一个协程并运行
async def print_message_async(message):
print("异步消息:", message)
loop = asyncio.get_event_loop()
loop.run_until_complete(print_message_async("Hello from async"))
loop.close()
```
这些示例展示了如何使用常用的 Python 标准库进行不同的操作,包括生成随机数、获取当前工作目录、处理时间和日期、正则表达式匹配、JSON序列化等。希望对您有所帮助!
Python 标准库中 datetime 类型转字符串
Python 标准库中的 datetime 类型可以使用 strftime() 方法将其转换为字符串。strftime() 方法接受一个格式化字符串作为参数,用于指定输出的格式。
例如,将当前时间转换为字符串,格式为 "年-月-日 时:分:秒",可以这样写:
```python
from datetime import datetime
now = datetime.now()
s = now.strftime("%Y-%m-%d %H:%M:%S")
print(s)
```
输出结果为:
```
2022-01-06 15:12:34
```
在格式化字符串中,各个占位符的含义如下:
- %Y:四位数年份
- %m:两位数月份
- %d:两位数日期
- %H:24 小时制小时数
- %M:分钟数
- %S:秒数
还可以使用其他占位符组合出不同的格式化字符串。需要注意的是,strftime() 方法返回的是一个字符串,而不是 datetime 对象。
阅读全文
相关推荐
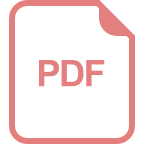
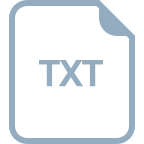
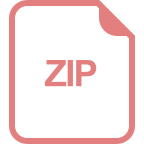













