随机生成彩色数字雨,且会根据屏幕大小生成不同密度的数字雨,使用代码python
时间: 2024-10-08 22:23:45 浏览: 26
在Python中,我们可以利用`pygame`库来创建一个随机的彩色数字雨效果,它会根据屏幕尺寸动态调整数字的数量和显示密度。下面是一个简单的例子:
```python
import pygame
import random
from datetime import datetime
# 初始化Pygame
pygame.init()
# 设置窗口大小和颜色模式
screen_width, screen_height = (800, 600)
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("Colorful Number Rain")
# 定义数字、颜色和字体
numbers = [str(i) for i in range(10)]
colors = [(random.randint(0, 255), random.randint(0, 255), random.randint(0, 255)) for _ in range(len(numbers))]
font = pygame.font.SysFont('Arial', 24)
# 游戏主循环
running = True
while running:
# 每秒生成新的数字
now = datetime.now()
dt_seconds = now.second % len(numbers)
num_to_show = numbers[dt_seconds]
# 根据屏幕宽度计算密度
density = min(screen_width // 100, 10)
# 创建并绘制数字
text_surface = font.render(num_to_show, True, colors[dt_seconds])
text_rect = text_surface.get_rect(center=(density * random.random() * screen_width, screen_height - 30))
screen.blit(text_surface, text_rect)
# 更新和检测事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN and event.key == pygame.K_ESCAPE:
running = False
# 更新屏幕
pygame.display.flip()
# 退出游戏
pygame.quit()
```
这个脚本会在屏幕上每秒钟生成一个新的随机彩色数字,数字的颜色和位置都是随机的,数字的密度随着屏幕宽度增加而降低,模拟了数字雨的效果。如果你想进一步调整它的外观,可以修改颜色、字体和密度的相关参数。
阅读全文
相关推荐
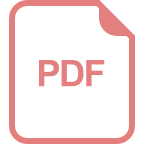
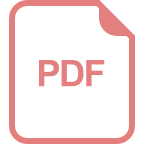
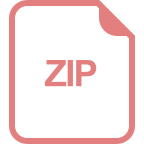

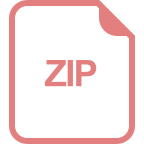
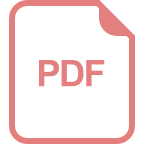
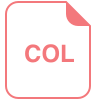
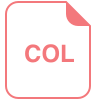
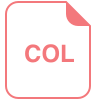
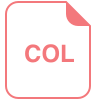
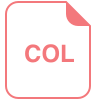
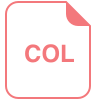
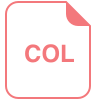
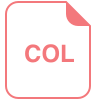
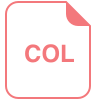
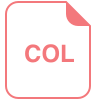
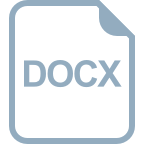