爬虫怎样将网页源码存入mysql
时间: 2024-09-28 07:15:09 浏览: 28
爬虫抓取网页源码并将其存储到MySQL数据库的过程通常包含以下几个步骤:
1. **网络请求**:首先,使用Python的`requests`库或其他类似工具发送HTTP GET请求获取网页内容。
```python
import requests
response = requests.get('http://example.com')
html_content = response.text
```
2. **解析HTML**:然后使用如BeautifulSoup、PyQuery或lxml等库对获取的HTML进行解析,提取需要的数据。
```python
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
data_to_store = soup.find_all('tag', class_='some_class') # 根据实际需要选择标签和属性
```
3. **数据清洗**:根据需求清洗和处理提取出的数据,例如去除特殊字符、转换格式等。
4. **连接数据库**:使用Python的`pymysql`或`sqlite3`等库连接MySQL服务器,并创建必要的表结构,如果还没有的话。
```python
import pymysql
conn = pymysql.connect(host='localhost', user='username', password='password', db='database_name')
cursor = conn.cursor()
```
5. **插入数据**:创建SQL插入语句,根据清洗后的数据逐条插入到MySQL表中。
```python
sql = "INSERT INTO table_name (column1, column2) VALUES (%s, %s)"
for item in data_to_store:
cursor.execute(sql, (item['field1'], item['field2']))
```
6. **提交事务和关闭连接**:最后记得提交事务并关闭数据库连接,以保证数据安全。
```python
conn.commit()
cursor.close()
conn.close()
```
相关推荐
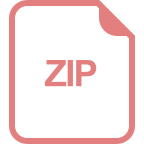
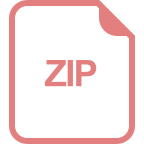
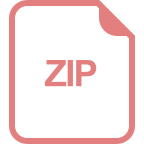














