针对二维坐标系上的点,定义一个点类,实现简单的数据输入输出
时间: 2024-04-29 13:21:23 浏览: 23
好的,下面是一个简单的点类的实现示例:
```python
class Point:
def __init__(self, x=0, y=0):
self.x = x
self.y = y
def input(self):
self.x = float(input("请输入点的 x 坐标:"))
self.y = float(input("请输入点的 y 坐标:"))
def output(self):
print("该点的坐标为:({:.2f}, {:.2f})".format(self.x, self.y))
```
这个点类有两个属性:x 和 y,分别表示点在二维坐标系上的横纵坐标。类中的 input 方法用于输入点的坐标,output 方法用于输出点的坐标。在 input 方法中,我们使用 float 函数将输入的字符串转换成浮点数类型。
使用示例:
```python
# 创建一个点对象
p = Point()
# 输入点的坐标
p.input()
# 输出点的坐标
p.output()
```
示例输出:
```
请输入点的 x 坐标:3.14
请输入点的 y 坐标:2.71
该点的坐标为:(3.14, 2.71)
```
相关问题
用禁忌搜索算法解决TSP问题,c++实现,每个节点用二维坐标系表示
好的,禁忌搜索算法可以用来解决TSP问题,以下是C++实现的示例代码:
```cpp
#include <iostream>
#include <vector>
#include <cmath>
#include <algorithm>
#include <ctime>
#include <cstdlib>
using namespace std;
const int MAXN = 100;
// 城市的数量
int n;
// 城市的坐标
int x[MAXN], y[MAXN];
// 最优路径和距离
vector<int> bestPath;
double bestDistance;
// 计算两个城市之间的距离
double dist(int i, int j) {
return sqrt((x[i]-x[j])*(x[i]-x[j]) + (y[i]-y[j])*(y[i]-y[j]));
}
// 计算一条路径的总距离
double getPathDistance(const vector<int>& path) {
double distance = 0.0;
for (int i = 0; i < n; ++i) {
distance += dist(path[i], path[(i+1)%n]);
}
return distance;
}
// 生成一个初始解
vector<int> generateInitialPath() {
vector<int> path(n);
for (int i = 0; i < n; ++i) {
path[i] = i;
}
random_shuffle(path.begin(), path.end());
return path;
}
// 交换路径中的两个城市
void swapCities(vector<int>& path, int i, int j) {
swap(path[i], path[j]);
}
// 禁忌搜索算法
void tabuSearch(int maxIter, int tabuSize) {
// 初始化当前路径和最优路径
vector<int> currentPath = generateInitialPath();
bestPath = currentPath;
bestDistance = getPathDistance(currentPath);
// 初始化禁忌表
vector<vector<int>> tabuList(n, vector<int>(n, 0));
for (int iter = 1; iter <= maxIter; ++iter) {
// 选择最好的相邻解
int bestI = -1, bestJ = -1;
double bestDelta = 1e9;
for (int i = 0; i < n; ++i) {
for (int j = i+1; j < n; ++j) {
// 计算交换位置i和j的路径长度变化
double delta = dist(currentPath[i], currentPath[j])
+ dist(currentPath[(i+1)%n], currentPath[(j+1)%n])
- dist(currentPath[i], currentPath[(i+1)%n])
- dist(currentPath[j], currentPath[(j+1)%n]);
// 如果这个交换比当前最好的还要好,且不在禁忌表中,就更新最好的交换位置
if (delta < bestDelta && tabuList[i][j] == 0) {
bestDelta = delta;
bestI = i;
bestJ = j;
}
}
}
// 更新当前路径和最优路径
swapCities(currentPath, bestI, bestJ);
double currentDistance = getPathDistance(currentPath);
if (currentDistance < bestDistance) {
bestPath = currentPath;
bestDistance = currentDistance;
}
// 将这个交换加入禁忌表
tabuList[bestI][bestJ] = tabuList[bestJ][bestI] = tabuSize;
for (int i = 0; i < n; ++i) {
for (int j = 0; j < n; ++j) {
if (tabuList[i][j] > 0) {
tabuList[i][j]--;
}
}
}
}
}
int main() {
srand(time(NULL));
// 输入城市的数量和坐标
cin >> n;
for (int i = 0; i < n; ++i) {
cin >> x[i] >> y[i];
}
// 运行禁忌搜索算法,输出最优路径和距离
tabuSearch(1000, n/2);
cout << "Best path:\n";
for (int i = 0; i < n; ++i) {
cout << bestPath[i] << " ";
}
cout << "\nBest distance: " << bestDistance << endl;
return 0;
}
```
这段代码中,我们定义了一个`tabuSearch()`函数来实现禁忌搜索算法,其中`maxIter`表示最大迭代次数,`tabuSize`表示禁忌表的大小。函数中首先生成一个初始路径,然后不断迭代,每次选择最好的相邻解并更新路径和禁忌表,直到达到最大迭代次数为止。最后输出最优路径和距离。
在`tabuSearch()`函数中,我们使用了一个二维数组`tabuList`来表示禁忌表,其中`tabuList[i][j]`表示位置i和j是否在禁忌表中。在每次更新路径和禁忌表时,我们将这个交换加入禁忌表,并将禁忌表中的所有交换次数减1,以保证交换不会一直在禁忌表中。
通过VScode 编写C++获取文本数据画一个二维图形
### 回答1:
好的,我可以帮助您。您可以通过在VScode中创建一个C文件来编写代码并读取文本数据。然后,您可以使用所读取的数据来创建一个二维图形。这涉及到C语言中的图形库,例如OpenGL或SDL。我可以提供一些基本的代码示例来帮助您入门。
### 回答2:
要通过VScode编写C语言程序来获取文本数据并画一个二维图形,可以按照以下步骤进行:
1. 首先,打开VScode,并确保已经安装了C语言的相关插件和编译器。
2. 创建一个新的C语言源文件,例如"main.c"。
3. 在源文件中,编写代码以读取文本数据。可以使用标准库函数如fopen()和fread()来打开和读取文件,或使用scanf()函数从标准输入读取数据。
4. 根据文本数据的格式和结构,解析数据并将其存储到适当的变量中。例如,如果文本数据是以一行一对坐标的形式给出,可以使用数组或链表存储这些坐标。
5. 使用图形库,如OpenGL或SDL,调用相关的函数以在图形窗口中绘制二维图形。例如,可以使用OpenGL的glBegin()和glVertex2f()函数来定义图形的形状和位置。
6. 遍历存储数据的变量,在图形窗口中逐个绘制点、线、多边形等。可以使用循环结构来访问每个数据点。
7. 最后,编译并运行程序。在VScode中使用编译器命令编译C文件,并确保没有错误和警告。运行程序后,应该能够在图形窗口中看到绘制的二维图形。
需要注意的是,具体的代码实现和绘图库的选择可能因个人情况而异。但以上步骤提供了一个基本的框架,可以根据具体需求进行调整和扩展。
### 回答3:
在VScode中编写C程序可以使用C标准库的函数和数据结构来获取文本数据,并以此数据为基础画出二维图形。
首先,在C程序中使用文件操作函数打开文本文件,使用文件读取函数将数据读入程序中。读取的数据可以是保存在文本文件中的数值,每个数值代表一个点或者一条线的坐标。
接下来,通过引入图形库,如OpenGL、SDL等,使用相关函数将读取到的数据绘制成二维图形。例如,在OpenGL中,可以使用glBegin()和glVertex2f()等函数设置绘图的起点和顶点坐标,再利用glEnd()函数结束绘图过程。可以使用循环语句来遍历读取到的所有数据,逐个绘制出相应的图形元素,以达到画出二维图形的效果。
在绘制图形之前,可以使用相关函数设置窗口大小、坐标系等参数,以保证绘制的图形可以正常显示。
最后,通过编译和运行C程序,即可在VScode的输出窗口中显示绘制好的二维图形。如果需要对图形进行交互操作,还可以加入相应的事件监听和处理函数,实现用户对图形的动态操作和控制。
总之,通过VScode 编写C程序并使用相关库函数,可以获取文本数据并绘制出二维图形,这是一种常见的数据可视化方法。
相关推荐
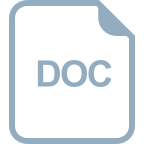













