java实现二叉树的创建和插入功能
时间: 2024-05-02 13:23:35 浏览: 147
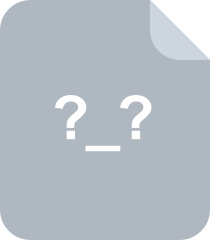
java实现二叉树
好的,关于二叉树的创建和插入功能,我可以为您提供以下的参考代码:
创建二叉树:
```java
public class TreeNode {
int val;
TreeNode left;
TreeNode right;
TreeNode(int x) { val = x; }
}
public class BinaryTree {
public static TreeNode createBinaryTree(int[] array) {
if (array == null || array.length == 0) {
return null;
}
TreeNode root = new TreeNode(array[0]);
Queue<TreeNode> queue = new LinkedList<>();
queue.offer(root);
int i = 1;
while (!queue.isEmpty()) {
TreeNode node = queue.poll();
if (i < array.length) {
if (array[i] != -1) {
node.left = new TreeNode(array[i]);
queue.offer(node.left);
}
i++;
}
if (i < array.length) {
if (array[i] != -1) {
node.right = new TreeNode(array[i]);
queue.offer(node.right);
}
i++;
}
}
return root;
}
}
```
插入节点:
```java
public class TreeNode {
int val;
TreeNode left;
TreeNode right;
TreeNode(int x) { val = x; }
}
public class BinaryTree {
public static void insertNode(TreeNode root, int val) {
if (root == null) {
root = new TreeNode(val);
return;
}
if (val < root.val) {
if (root.left == null) {
root.left = new TreeNode(val);
} else {
insertNode(root.left, val);
}
} else {
if (root.right == null) {
root.right = new TreeNode(val);
} else {
insertNode(root.right, val);
}
}
}
}
```
您可以根据自己的需要进行调整和修改。希望能够帮到您!
阅读全文
相关推荐
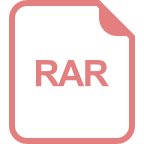

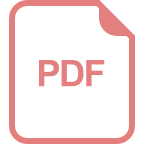
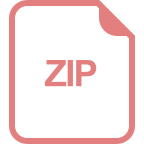
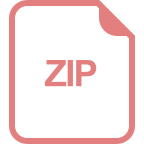
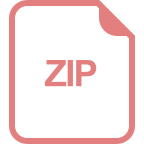
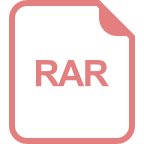
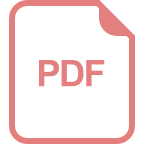
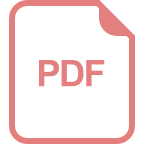
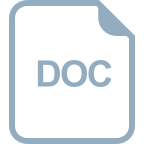
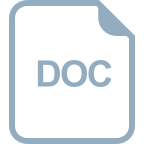
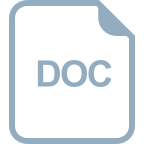
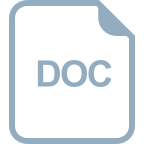
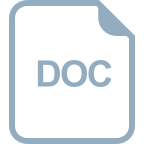
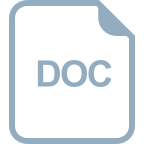

