使用java实现二叉树
时间: 2023-06-17 22:05:56 浏览: 53
二叉树是一种非常常见的数据结构,它由一个根节点和最多两个子节点组成,每个子节点也可以再有两个子节点,以此类推。下面是一个使用Java实现二叉树的示例代码。
```java
public class Node {
int data;
Node left, right;
public Node(int item) {
data = item;
left = right = null;
}
}
public class BinaryTree {
Node root;
public BinaryTree(int key) {
root = new Node(key);
}
public BinaryTree() {
root = null;
}
public void insert(int key) {
root = insert(root, key);
}
private Node insert(Node root, int key) {
if (root == null) {
root = new Node(key);
return root;
}
if (key < root.data)
root.left = insert(root.left, key);
else if (key > root.data)
root.right = insert(root.right, key);
return root;
}
public void inorder() {
inorder(root);
}
private void inorder(Node root) {
if (root != null) {
inorder(root.left);
System.out.print(root.data + " ");
inorder(root.right);
}
}
public static void main(String[] args) {
BinaryTree tree = new BinaryTree();
tree.insert(50);
tree.insert(30);
tree.insert(20);
tree.insert(40);
tree.insert(70);
tree.insert(60);
tree.insert(80);
tree.inorder();
}
}
```
在这个示例中,我们定义了一个`Node`类表示二叉树的节点,包括一个整型数据`data`和左右子节点`left`和`right`。我们还定义了一个`BinaryTree`类表示二叉树,包括一个根节点`root`和一些操作方法,如插入节点`insert`和中序遍历`inorder`。
在`insert`方法中,我们使用递归来插入新的节点。如果当前节点为`null`,则创建一个新节点。否则,将新节点与当前节点进行比较,如果小于当前节点,则插入到左子树中,否则插入到右子树中。最后,返回当前节点。
在`inorder`方法中,我们使用递归来进行中序遍历,即先遍历左子树,再访问当前节点,最后遍历右子树。在主方法中,我们创建一个二叉树并插入一些节点,然后进行中序遍历输出结果。
相关推荐
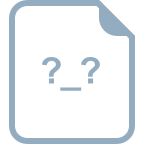














