使用mybatisplus生成一个学生管理系统
时间: 2023-07-13 07:26:09 浏览: 56
好的,我可以为您提供一些基本的步骤:
1. 创建数据库
首先,您需要创建一个数据库,例如 `student_db`,并在其中创建一个名为 `student` 的表,该表包含以下字段:`id`、`name`、`age`、`gender`、`class`。
2. 添加依赖
在 `pom.xml` 中添加 Mybatis-Plus 的依赖:
```xml
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>x.x.x</version>
</dependency>
```
其中,`x.x.x` 为 Mybatis-Plus 的版本号。
3. 创建实体类
创建一个名为 `Student` 的实体类,该类包含与表中字段相对应的属性以及 getter 和 setter 方法。
```java
@Data
public class Student {
private Long id;
private String name;
private Integer age;
private String gender;
private String className;
}
```
4. 创建 Mapper 接口
创建一个名为 `StudentMapper` 的 Mapper 接口,该接口继承 `BaseMapper` 接口,并指定泛型为 `Student`,这样就可以使用 Mybatis-Plus 提供的通用 CRUD 方法。
```java
public interface StudentMapper extends BaseMapper<Student> {
}
```
5. 配置 application.yml
在 `application.yml` 中配置数据库连接信息以及 Mybatis-Plus 的相关配置。
```yaml
spring:
datasource:
driver-class-name: com.mysql.jdbc.Driver
url: jdbc:mysql://localhost:3306/student_db?useSSL=false&useUnicode=true&characterEncoding=UTF-8
username: root
password: root
mybatis-plus:
mapper-locations: classpath:mapper/*.xml
typeAliasesPackage: com.example.demo.entity
```
6. 编写 Service 层和 Controller 层
创建一个名为 `StudentService` 的 Service 接口,并实现相关的业务逻辑。
```java
public interface StudentService extends IService<Student> {
}
```
创建一个名为 `StudentServiceImpl` 的 Service 实现类,实现 `StudentService` 接口。
```java
@Service
public class StudentServiceImpl extends ServiceImpl<StudentMapper, Student> implements StudentService {
}
```
创建一个名为 `StudentController` 的 Controller 类,实现相关的接口,并注入 `StudentService`。
```java
@RestController
@RequestMapping("/student")
public class StudentController {
@Autowired
private StudentService studentService;
@GetMapping("/{id}")
public Student getStudentById(@PathVariable Long id) {
return studentService.getById(id);
}
@PostMapping("/")
public boolean saveStudent(@RequestBody Student student) {
return studentService.save(student);
}
@PutMapping("/")
public boolean updateStudent(@RequestBody Student student) {
return studentService.updateById(student);
}
@DeleteMapping("/{id}")
public boolean deleteStudentById(@PathVariable Long id) {
return studentService.removeById(id);
}
}
```
7. 编写 SQL 语句
在 `resources/mapper` 目录下创建一个名为 `StudentMapper.xml` 的文件,编写相关的 SQL 语句。
```xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.example.demo.mapper.StudentMapper">
<resultMap id="BaseResultMap" type="com.example.demo.entity.Student">
<id column="id" property="id" />
<result column="name" property="name" />
<result column="age" property="age" />
<result column="gender" property="gender" />
<result column="class_name" property="className" />
</resultMap>
<select id="selectById" resultMap="BaseResultMap">
select * from student where id = #{id}
</select>
<insert id="insert" parameterType="com.example.demo.entity.Student">
insert into student(name, age, gender, class_name)
values(#{name}, #{age}, #{gender}, #{className})
</insert>
<update id="update" parameterType="com.example.demo.entity.Student">
update student set name=#{name}, age=#{age}, gender=#{gender}, class_name=#{className}
where id=#{id}
</update>
<delete id="deleteById" parameterType="java.lang.Long">
delete from student where id=#{id}
</delete>
</mapper>
```
这样就完成了一个简单的基于 Mybatis-Plus 的学生管理系统。
相关推荐
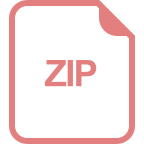















