c语言实现:输入包含两行: 第一行是第一个“时:分:秒”格式的时间。 第二行是第二个“时:分:秒”格式的时间。 且本题保证第一个时间一定大于第二个时间! 输出两个时间之间的秒数差。
时间: 2024-09-23 07:06:13 浏览: 24
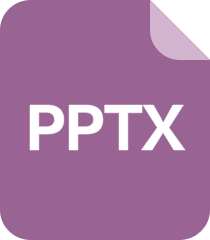
C语言第07章-一维数组和二维数组1完整.pptx
在C语言中,我们可以创建一个程序来处理这个问题,但需要注意的是,由于C标准库并不直接提供解析字符串为特定格式的时间的功能,我们需要自定义一些函数来分割和转换时间字符串。以下是一个简单的实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdbool.h>
// 将字符串按冒号拆分成整数数组
void split_time(char* str, int time[], int n) {
char* token = strtok(str, ":");
for (int i = 0; i < n && token != NULL; ++i) {
time[i] = atoi(token);
token = strtok(NULL, ":");
}
}
// 比较两个时间数组,返回秒数差
int compare_times(int time1[], int time2[]) {
int diff_hours = time1[0] - time2[0];
int diff_mins = time1[1] - time2[1];
int diff_secs = time1[2] - time2[2];
// 超过24小时的部分需要减去一天的总秒数
if (diff_hours < 0 || (diff_hours == 0 && diff_mins < 0)) {
diff_hours += 24;
diff_mins -= 60;
diff_secs += 3600;
}
return diff_secs + diff_mins * 60 + diff_hours * 3600;
}
int main() {
char str1[20], str2[20];
int time1[3], time2[3];
// 读取两个时间
fgets(str1, sizeof(str1), stdin);
fgets(str2, sizeof(str2), stdin);
// 去除末尾换行符
str1[strlen(str1) - 1] = '\0';
str2[strlen(str2) - 1] = '\0';
// 分割时间字符串
split_time(str1, time1, 3);
split_time(str2, time2, 3);
// 检查第一个时间是否大于第二个
if (!compare_times(time1, time2)) {
printf("错误:第一个时间不应小于第二个。\n");
return 1;
}
// 计算秒数差并输出
int sec_diff = compare_times(time1, time2);
printf("两个时间之间的秒数差为: %d 秒.\n", sec_diff);
return 0;
}
```
这个程序首先读取两个时间字符串,然后使用`split_time`函数将其分割成小时、分钟和秒三个部分。接下来,比较这两个时间并计算秒数差。如果第一个时间小于第二个,程序会显示错误信息并退出。
阅读全文
相关推荐
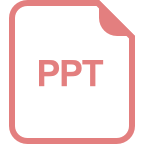
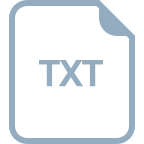















