安卓进行post请求,发送自定义cookie
时间: 2023-08-31 12:25:07 浏览: 47
### 回答1:
安卓进行 post 请求,发送自定义 cookie 的方法如下:
1. 首先,在 Android 中使用 HTTP 请求,推荐使用第三方库例如 OkHttp 或者 Volley。
2. 使用 OkHttp,你可以使用如下代码创建带有自定义 cookie 的请求:
```
OkHttpClient client = new OkHttpClient();
String cookie = "key=value";
Request request = new Request.Builder()
.url(url)
.post(requestBody)
.addHeader("Cookie", cookie)
.build();
Response response = client.newCall(request).execute();
```
3. 使用 Volley,你可以使用如下代码创建带有自定义 cookie 的请求:
```
RequestQueue queue = Volley.newRequestQueue(context);
StringRequest stringRequest = new StringRequest(Request.Method.POST, url,
new Response.Listener<String>() {
@Override
public void onResponse(String response) {
// 处理响应
}
},
new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
// 处理错误
}
}) {
@Override
public Map<String, String> getHeaders() throws AuthFailureError {
Map<String, String> headers = new HashMap<>();
String cookie = "key=value";
headers.put("Cookie", cookie);
return headers;
}
@Override
protected Map<String, String> getParams() throws AuthFailureError {
// 设置请求参数
Map<String, String> params = new HashMap<>();
// ...
return params;
}
};
queue.add(stringRequest);
```
注意,上面的代码假设 cookie 的格式是 "key=value",如果你有多个 cookie,需要将它们拼接在一起,例如 "key1=value1; key2=value2"。
### 回答2:
在安卓设备上进行POST请求并发送自定义的Cookie,可以通过使用Java代码和HttpClient库来实现。
首先,需要在AndroidManifest.xml文件中添加网络权限:
```xml
<uses-permission android:name="android.permission.INTERNET" />
```
然后,在项目的build.gradle文件中添加HttpClient库的依赖:
```groovy
dependencies {
implementation 'org.apache.httpcomponents:httpclient:4.5.13'
}
```
接下来,在代码中进行POST请求的逻辑。可以创建一个名为"HttpPostRequest"的类,并在该类中编写发送POST请求的方法:
```java
import org.apache.http.HttpResponse;
import org.apache.http.HttpEntity;
import org.apache.http.NameValuePair;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.util.EntityUtils;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.impl.client.DefaultHttpClient;
import java.util.ArrayList;
import java.util.List;
public class HttpPostRequest {
private static final String URL = "YOUR_POST_URL";
public static String sendPostRequestWithCookie(String postData, String cookieValue) {
HttpClient httpClient = new DefaultHttpClient();
HttpPost httpPost = new HttpPost(URL);
try {
// 设置自定义Cookie
httpPost.addHeader("Cookie", "your-cookie-name=" + cookieValue);
// 设置POST请求参数
List<NameValuePair> params = new ArrayList<>();
params.add(new BasicNameValuePair("data", postData));
httpPost.setEntity(new UrlEncodedFormEntity(params, "UTF-8"));
// 发送POST请求
HttpResponse httpResponse = httpClient.execute(httpPost);
HttpEntity httpEntity = httpResponse.getEntity();
// 获取响应结果
String response = EntityUtils.toString(httpEntity);
return response;
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
}
```
以上代码示例中,URL为你要发送POST请求的地址,在addHeader方法中使用自定义的Cookie名称和值,设置POST请求参数,并执行请求。最后,可以获取到响应结果。
需要注意的是,HttpClient库从Android 6.0(API级别23)开始被弃用,推荐使用HttpURLConnection或OkHttp库来代替。
### 回答3:
安卓进行POST请求时,可以通过设置请求头来发送自定义cookie。以下是一个示例代码:
```java
// 导入所需的包
import java.io.BufferedReader;
import java.io.DataOutputStream;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
// 定义发送POST请求的方法
private String sendPostRequest(String url, String cookie, String postData) {
StringBuilder response = new StringBuilder();
try {
// 创建URL对象
URL obj = new URL(url);
// 打开连接
HttpURLConnection conn = (HttpURLConnection) obj.openConnection();
// 设置请求方法为POST
conn.setRequestMethod("POST");
// 设置请求头的cookie
conn.setRequestProperty("Cookie", cookie);
// 设置其他必要的请求头参数
// 发送POST请求参数,如果有的话
if (postData != null) {
conn.setDoOutput(true);
DataOutputStream wr = new DataOutputStream(conn.getOutputStream());
wr.writeBytes(postData);
wr.flush();
wr.close();
}
// 获取响应结果
BufferedReader in = new BufferedReader(new InputStreamReader(conn.getInputStream()));
String inputLine;
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
} catch (Exception e) {
e.printStackTrace();
}
return response.toString();
}
// 调用示例
String url = "http://example.com/api";
String cookie = "your_custom_cookie=123456789";
String postData = "param1=value1¶m2=value2";
String response = sendPostRequest(url, cookie, postData);
```
在上述示例中,通过设置请求头的Cookie属性,将自定义的cookie值发送到服务器。根据实际需要,可以进一步设置其他请求头参数,如User-Agent等。发送POST请求时,可以传递需要发送的参数,通过DataOutputStream写入请求体中。最后,通过读取连接的输入流获取服务器返回的响应结果。
相关推荐
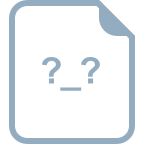
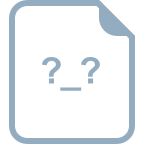
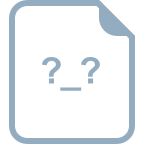
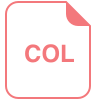
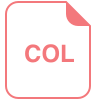
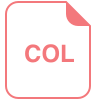
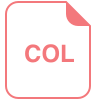
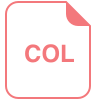









