用python写一个神经网络学习的模型,数据使用随机数据
时间: 2023-05-21 17:01:08 浏览: 83
好的,以下是一个简单的用 Python 编写的神经网络学习模型,使用随机数据进行训练:
```python
import numpy as np
# 定义神经网络模型
class NeuralNetwork:
def __init__(self):
# 随机初始化权重
self.weights = np.random.rand(3, 1)
def sigmoid(self, x):
# sigmoid 激活函数
return 1 / (1 + np.exp(-x))
def sigmoid_derivative(self, x):
# sigmoid 激活函数的导数
return x * (1 - x)
def train(self, inputs, outputs, iterations):
for i in range(iterations):
# 前向传播
predicted_output = self.predict(inputs)
# 计算误差
error = outputs - predicted_output
# 反向传播
adjustment = np.dot(inputs.T, error * self.sigmoid_derivative(predicted_output))
self.weights += adjustment
def predict(self, inputs):
# 前向传播
return self.sigmoid(np.dot(inputs, self.weights))
# 随机生成训练数据
inputs = np.random.rand(100, 3)
outputs = np.random.rand(100, 1)
# 创建神经网络模型并进行训练
neural_network = NeuralNetwork()
neural_network.train(inputs, outputs, 1000)
# 使用训练好的模型进行预测
test_input = np.array([0.5, 0.5, 0.5])
predicted_output = neural_network.predict(test_input)
print(predicted_output)
```
这个模型使用了一个简单的三层神经网络,其中输入层有三个神经元,输出层有一个神经元。训练数据使用了随机生成的数据,训练过程中使用了梯度下降算法进行优化。最后,我们使用训练好的模型对一个新的输入进行预测。
相关推荐
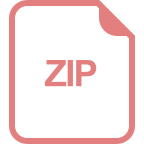
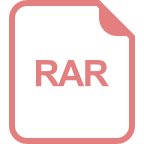














